Start GDB on your project.
Go to project directory, where you've already compiled the project executable. Issue the command gdb and the name of the executable as below:
gdb projectExecutablename
This starts up gdb, prints the following: GNU gdb (Ubuntu 7.11.1-0ubuntu1~16.04) 7.11.1 Copyright (C) 2016 Free Software Foundation, Inc. ................................................. Type "apropos word" to search for commands related to "word"... Reading symbols from projectExecutablename...done. (gdb)
Before you start your program running, you want to set up your breakpoints. The break command allows you to do so. To set a breakpoint at the beginning of the function named main:
(gdb) b main
Once you've have the (gdb) prompt, the run command starts the executable running. If the program you are debugging requires any command-line arguments, you specify them to the run command. If you wanted to run my program on the "xfiles" file (which is in a folder "mulder" in the project directory), you'd do the following:
(gdb) r mulder/xfiles
Hope this helps.
Disclaimer: This solution is not mine, it is adapted from https://web.stanford.edu/class/cs107/guide_gdb.html This short guide to gdb was, most probably, developed at Stanford University.
The accepted answer is correct, but I found another cause if you're developing under ASP.NET with Visual Studio 2013 or higher and are sure you didn't make any synchronous ajax requests or define any scripts in the wrong place.
The solution is to disable the "Browser Link" feature by unchecking "Enable Browser Link" in the VS toolbar dropdown indicated by the little refresh icon pointing clockwise. As soon as you do this and reload the page, the warnings should stop!
This should only happen while debugging locally, but it's still nice to know the cause of the warnings.
Okay, here's a list of things to look into:
1) If you're not running a webserver of any kind and just testing with file://index.html, then you're probably running into same-origin policy issues. See:
https://code.google.com/archive/p/browsersec/wikis/Part2.wiki#Same-origin_policy
Many browsers don't allow locally hosted files to access other locally hosted files. Firefox does allow it, but only if the file you're loading is contained in the same folder as the html file (or a subfolder).
2) The success function returned from $http.get() already splits up the result object for you:
$http({method: 'GET', url: '/someUrl'}).success(function(data, status, headers, config) {
So it's redundant to call success with function(response) and return response.data.
3) The success function does not return the result of the function you pass it, so this does not do what you think it does:
var mainInfo = $http.get('content.json').success(function(response) {
return response.data;
});
This is closer to what you intended:
var mainInfo = null;
$http.get('content.json').success(function(data) {
mainInfo = data;
});
4) But what you really want to do is return a reference to an object with a property that will be populated when the data loads, so something like this:
theApp.factory('mainInfo', function($http) {
var obj = {content:null};
$http.get('content.json').success(function(data) {
// you can do some processing here
obj.content = data;
});
return obj;
});
mainInfo.content will start off null, and when the data loads, it will point at it.
Alternatively you can return the actual promise the $http.get returns and use that:
theApp.factory('mainInfo', function($http) {
return $http.get('content.json');
});
And then you can use the value asynchronously in calculations in a controller:
$scope.foo = "Hello World";
mainInfo.success(function(data) {
$scope.foo = "Hello "+data.contentItem[0].username;
});
Was able to solve this problem in my asp.net mvc project by updating my version of Newton.Json (old Version = 9.0.0.0 to new Version 11.0.0.0) usign Package Manager.
The function call operator.
class Foo:
def __call__(self, a, b, c):
# do something
x = Foo()
x(1, 2, 3)
The __call__ method can be used to redefined/re-initialize the same object. It also facilitates the use of instances/objects of a class as functions by passing arguments to the objects.
I solved this by opening the plugin in settings, where under the 'installed' tab, I noticed the blue text 'Plugin homepage' which was a shortcut to the JetBrains plugins. There was an agreement which I had to accept to get the full functionality. I did accept and I also edited my environment variables by adding path to the bin of dart-SDK. Previously I only had the bin of flutter added to path. Anyway, this solved my problem.
if (drMyRow.Table.Columns["ColNameToCheck"] != null)
{
doSomethingUseful;
{
else { return; }
Although the DataRow does not have a Columns property, it does have a Table that the column can be checked for.
Result Set
are actually contains multiple rows of data, and use a cursor to point out current position. So in your case, rs4.getString(1)
only get you the data in first column of first row. In order to change to next row, you need to call next()
a quick example
while (rs.next()) {
String sid = rs.getString(1);
String lid = rs.getString(2);
// Do whatever you want to do with these 2 values
}
there are many useful method in ResultSet
, you should take a look :)
This can be solved by the following step:
Please ensure "Windows Hypervisor Platform" is installed. If it's not installed, install it, restart your computer and you will be good to go.
This is a perfect example of where you should use a javascript library like Prototype or JQuery to abstract away the cross-browser differences.
Another way of writing <script>window.$ = window.jQuery = require('./path/to/jquery');</script>
is :
<script src="./path/to/jquery" onload="window.$ = window.jQuery = module.exports;"></script>
You have to create two triggers, but you can move the common code into a procedure and have them both call the procedure.
Upon installation, the Android SDK generates a debug
signing certificate for you in a keystore called debug.keystore
. The Eclipse plug-in uses this certificate to sign each application build that is generated.
Unfortunately a debug certificate is only valid for 365 days. To generate a new one you must delete the existing debug.keystore
file. Its location is platform dependent - you can find it in Preferences - Android - Build - Default debug keystore.
You can solve this problem by actually unchecking the IIS tools in your Windows feature list. Then, repair your Visual Studio 2013 installation and make sure Web Developer is checked. It will install IIS 8 with which VS will work nicely.
Sign in and the 30 day trial will go away!
"And if you're already signed in, sign out then sign in again." –b1nary.atr0phy
If only names of regular files immediately contained within a directory (assume it's ~/dirs
) are needed, you can do
find ~/docs -type f -maxdepth 1 > filenames.txt
You can use functions in pyspark.sql.functions
: functions like year
, month
, etc
refer to here: https://spark.apache.org/docs/latest/api/python/pyspark.sql.html#pyspark.sql.DataFrame
from pyspark.sql.functions import *
newdf = elevDF.select(year(elevDF.date).alias('dt_year'), month(elevDF.date).alias('dt_month'), dayofmonth(elevDF.date).alias('dt_day'), dayofyear(elevDF.date).alias('dt_dayofy'), hour(elevDF.date).alias('dt_hour'), minute(elevDF.date).alias('dt_min'), weekofyear(elevDF.date).alias('dt_week_no'), unix_timestamp(elevDF.date).alias('dt_int'))
newdf.show()
+-------+--------+------+---------+-------+------+----------+----------+
|dt_year|dt_month|dt_day|dt_dayofy|dt_hour|dt_min|dt_week_no| dt_int|
+-------+--------+------+---------+-------+------+----------+----------+
| 2015| 9| 6| 249| 0| 0| 36|1441497601|
| 2015| 9| 6| 249| 0| 0| 36|1441497601|
| 2015| 9| 6| 249| 0| 0| 36|1441497603|
| 2015| 9| 6| 249| 0| 1| 36|1441497694|
| 2015| 9| 6| 249| 0| 20| 36|1441498808|
| 2015| 9| 6| 249| 0| 20| 36|1441498811|
| 2015| 9| 6| 249| 0| 20| 36|1441498815|
I have found that at times even verifying the settings under Options --> Statement Completion (the answer above) doesn't work. In this case, saving and restarting Visual Studio will re-enable Intellisense.
Finally, this link has a list of other ways to troubleshoot Intellisense, broken down by language (for more specific errors).
http://msdn.microsoft.com/en-us/library/vstudio/ecfczya1(v=vs.100).aspx
$('input:checkbox:checked').val(); // get the value from a checked checkbox
As an extension of @Demyn's answer, I created some utility functions which print the current function's name and current function's arguments:
import inspect
import logging
import traceback
def get_function_name():
return traceback.extract_stack(None, 2)[0][2]
def get_function_parameters_and_values():
frame = inspect.currentframe().f_back
args, _, _, values = inspect.getargvalues(frame)
return ([(i, values[i]) for i in args])
def my_func(a, b, c=None):
logging.info('Running ' + get_function_name() + '(' + str(get_function_parameters_and_values()) +')')
pass
logger = logging.getLogger()
handler = logging.StreamHandler()
formatter = logging.Formatter(
'%(asctime)s [%(levelname)s] -> %(message)s')
handler.setFormatter(formatter)
logger.addHandler(handler)
logger.setLevel(logging.INFO)
my_func(1, 3) # 2016-03-25 17:16:06,927 [INFO] -> Running my_func([('a', 1), ('b', 3), ('c', None)])
I don't know if this will help anybody, but my problem was I was trying to set the gradient to the "src" property of an ImageView like so:
<ImageView
android:id="@+id/imgToast"
android:layout_width="wrap_content"
android:layout_height="60dp"
android:src="@drawable/toast_bg"
android:adjustViewBounds="true"
android:scaleType="fitXY"/>
Not 100% sure why that didn't work, but now I changed it and put the drawable in the "background" property of the ImageView's parent, which is a RelativeLayout in my case, like so: (this worked successfully)
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:id="@+id/custom_toast_layout_id"
android:layout_height="match_parent"
android:background="@drawable/toast_bg">
Safest way :
static_cast<int>(reinterpret_cast<long>(void * your_variable));
long
guarantees a pointer size on Linux on any machine. Windows has 32 bit long only on 64 bit as well. Therefore, you need to change it to long long
instead of long
in windows for 64 bits.
So reinterpret_cast
has casted it to long
type and then static_cast
safely casts long
to int
, if you are ready do truncte the data.
From matplotlib 3.1 onwards you may use ax.secondary_xaxis
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(1,13, num=301)
y = (np.sin(x)+1.01)*3000
# Define function and its inverse
f = lambda x: 1/(1+x)
g = lambda x: 1/x-1
fig, ax = plt.subplots()
ax.semilogy(x, y, label='DM')
ax2 = ax.secondary_xaxis("top", functions=(f,g))
ax2.set_xlabel("1/(x+1)")
ax.set_xlabel("x")
plt.show()
I've tried different ways of blocking scrolling when the arrow keys are pressed, both jQuery and native Javascript - they all work fine in Firefox, but don't work in recent versions of Chrome.
Even the explicit {passive: false}
property for window.addEventListener
, which is recommended as the only working solution, for example here.
In the end, after many tries, I found a way that works for me in both Firefox and Chrome:
window.addEventListener('keydown', (e) => {
if (e.target.localName != 'input') { // if you need to filter <input> elements
switch (e.keyCode) {
case 37: // left
case 39: // right
e.preventDefault();
break;
case 38: // up
case 40: // down
e.preventDefault();
break;
default:
break;
}
}
}, {
capture: true, // this disables arrow key scrolling in modern Chrome
passive: false // this is optional, my code works without it
});
Quote for EventTarget.addEventListener()
from MDN
options Optional
An options object specifies characteristics about the event listener. The available options are:capture
ABoolean
indicating that events of this type will be dispatched to the registeredlistener
before being dispatched to anyEventTarget
beneath it in the DOM tree.
once
...
passive
ABoolean
that, if true, indicates that the function specified bylistener
will never callpreventDefault()
. If a passive listener does callpreventDefault()
, the user agent will do nothing other than generate a console warning. ...
This works for me:
$.get("data.php", function(data){
var expected = ['justIn', 'recent', 'old'];
var outString = '';
$.each(expected, function(i, val){
var contentArray = data[val];
outString += '<ul><li><b>' + val + '</b>: ';
$.each(contentArray, function(i1, val2){
var textID = val2.textId;
var text = val2.text;
var textType = val2.textType;
outString += '<br />('+textID+') '+'<i>'+text+'</i> '+textType;
});
outString += '</li></ul>';
});
$('#contentHere').append(outString);
}, 'json');
This produces this output:
<div id="contentHere"><ul>
<li><b>justIn</b>:
<br />
(123) <i>Hello</i> Greeting<br>
(514) <i>What's up?</i> Question<br>
(122) <i>Come over here</i> Order</li>
</ul><ul>
<li><b>recent</b>:
<br />
(1255) <i>Hello</i> Greeting<br>
(6564) <i>What's up?</i> Question<br>
(0192) <i>Come over here</i> Order</li>
</ul><ul>
<li><b>old</b>:
<br />
(5213) <i>Hello</i> Greeting<br>
(9758) <i>What's up?</i> Question<br>
(7655) <i>Come over here</i> Order</li>
</ul></div>
And looks like this:
Also, remember to set the contentType
as 'json'
HTML
<div id='a'>
<div>
<a class='click'>abc</a>
</div>
</div>
You could use the XPATH as :
//div[@id='a']//a[@class='click']
output
<a class="click">abc</a>
That said your Python code should be as :
driver.find_element_by_xpath("//div[@id='a']//a[@class='click']")
You can use Addressable::URI
gem for that:
require 'addressable/uri'
string = '\x12\x34\x56\x78\x9a\xbc\xde\xf1\x23\x45\x67\x89\xab\xcd\xef\x12\x34\x56\x78\x9a'
Addressable::URI.encode_component(string, Addressable::URI::CharacterClasses::QUERY)
# "%5Cx12%5Cx34%5Cx56%5Cx78%5Cx9a%5Cxbc%5Cxde%5Cxf1%5Cx23%5Cx45%5Cx67%5Cx89%5Cxab%5Cxcd%5Cxef%5Cx12%5Cx34%5Cx56%5Cx78%5Cx9a"
It uses more modern format, than CGI.escape
, for example, it properly encodes space as %20
and not as +
sign, you can read more in "The application/x-www-form-urlencoded type" on Wikipedia.
2.1.2 :008 > CGI.escape('Hello, this is me')
=> "Hello%2C+this+is+me"
2.1.2 :009 > Addressable::URI.encode_component('Hello, this is me', Addressable::URI::CharacterClasses::QUERY)
=> "Hello,%20this%20is%20me"
Doing the following in a command prompt works for me, also adding to my User environment variables worked fine as well:
set PATH=%PATH%;C:\Program Files\7-Zip\
echo %PATH%
7z
You should see as output (or something similar - as this is on my laptop running Windows 7):
C:\Users\Phillip>set PATH=%PATH%;C:\Program Files\7-Zip\
C:\Users\Phillip>echo %PATH%
C:\Program Files\Common Files\Microsoft Shared\Windows Live;C:\Program Files (x86)\NVIDIA Corporation\PhysX\Common;C:\Wi
ndows\system32;C:\Windows;C:\Windows\System32\Wbem;C:\Program Files\Intel\WiFi\bin\;C:\Program Files\Common Files\Intel\
WirelessCommon\;C:\Program Files (x86)\Microsoft SQL Server\100\Tools\Binn\;C:\Program Files\Microsoft SQL Server\100\To
ols\Binn\;C:\Program Files\Microsoft SQL Server\100\DTS\Binn\;C:\Windows\System32\WindowsPowerShell\v1.0\;C:\Program Fil
es (x86)\QuickTime\QTSystem\;C:\Program Files\Common Files\Microsoft Shared\Windows Live;C:\Program Files (x86)\Notepad+
+;C:\Program Files\Intel\WiFi\bin\;C:\Program Files\Common Files\Intel\WirelessCommon\;C:\Program Files\7-Zip\
C:\Users\Phillip>7z
7-Zip [64] 9.20 Copyright (c) 1999-2010 Igor Pavlov 2010-11-18
Usage: 7z <command> [<switches>...] <archive_name> [<file_names>...]
[<@listfiles...>]
<Commands>
a: Add files to archive
b: Benchmark
d: Delete files from archive
e: Extract files from archive (without using directory names)
l: List contents of archive
t: Test integrity of archive
u: Update files to archive
x: eXtract files with full paths
<Switches>
-ai[r[-|0]]{@listfile|!wildcard}: Include archives
-ax[r[-|0]]{@listfile|!wildcard}: eXclude archives
-bd: Disable percentage indicator
-i[r[-|0]]{@listfile|!wildcard}: Include filenames
-m{Parameters}: set compression Method
-o{Directory}: set Output directory
-p{Password}: set Password
-r[-|0]: Recurse subdirectories
-scs{UTF-8 | WIN | DOS}: set charset for list files
-sfx[{name}]: Create SFX archive
-si[{name}]: read data from stdin
-slt: show technical information for l (List) command
-so: write data to stdout
-ssc[-]: set sensitive case mode
-ssw: compress shared files
-t{Type}: Set type of archive
-u[-][p#][q#][r#][x#][y#][z#][!newArchiveName]: Update options
-v{Size}[b|k|m|g]: Create volumes
-w[{path}]: assign Work directory. Empty path means a temporary directory
-x[r[-|0]]]{@listfile|!wildcard}: eXclude filenames
-y: assume Yes on all queries
<!DOCTYPE html>
<html>
<head></head>
<body>
<div style=" background-color: #00000088"> Hi there </div>
<!-- #00 would be r, 00 would be g, 00 would be b, 88 would be a. -->
</body>
</html>
including 4 sets of numbers would make it rgba, not cmyk, but either way would work (rgba= 00000088, cmyk= 0%, 0%, 0%, 50%)
I think you are converting the data a bit more than you need to. Once you create the buffer with the proper encoding, you just need to write the buffer to the file.
var base64Data = req.rawBody.replace(/^data:image\/png;base64,/, "");
require("fs").writeFile("out.png", base64Data, 'base64', function(err) {
console.log(err);
});
new Buffer(..., 'base64') will convert the input string to a Buffer, which is just an array of bytes, by interpreting the input as a base64 encoded string. Then you can just write that byte array to the file.
As mentioned in the comments, req.rawBody
is no longer a thing. If you are using express
/connect
then you should use the bodyParser()
middleware and use req.body
, and if you are doing this using standard Node then you need to aggregate the incoming data
event Buffer
objects and do this image data parsing in the end
callback.
update-alternatives
is problematic in this case as it forces you to update all the elements depending on the JDK.
For this specific purpose, the package java-common
contains a tool called update-java-alternatives
.
It's straightforward to use it. First list the JDK installs available on your machine:
root@mylaptop:~# update-java-alternatives -l
java-1.7.0-openjdk-amd64 1071 /usr/lib/jvm/java-1.7.0-openjdk-amd64
java-1.8.0-openjdk-amd64 1069 /usr/lib/jvm/java-1.8.0-openjdk-amd64
And then pick one up:
root@mylaptop:~# update-java-alternatives -s java-1.7.0-openjdk-amd64
In addition, if you run a HTML document with multiple <tbody>
tags through W3C's HTML Validator, with a HTML5 DOCTYPE, it will successfully validate.
Wildcards aren't expanded inside quoted strings. And when wildcard is expanded, it's returned unchanged if there are no matches, it doesn't expand into an empty string. Try:
output="$(ls home/edward/bank1/fiche/Test* 2>/dev/null)"
if [ -n "$output" ]
then echo "Found one"
else echo "Found none"
fi
If the wildcard expanded to filenames, ls
will list them on stdout
; otherwise it will print an error on stderr
, and nothing on stdout. The contents of stdout
are assigned to output
.
if [ -n "$output" ]
tests whether $output
contains anything.
Another way to write this would be:
if [ $(ls home/edward/bank1/fiche/Test* 2>/dev/null | wc -l) -gt 0 ]
You may make it work without modifiying the server by making the broswer including the header Access-Control-Allow-Origin: *
in the HTTP OPTIONS' responses.
In Chrome, use this extension. If you are on Mozilla check this answer.
Try this,
HtmlElement head = _windowManager.ActiveBrowser.Document.GetElementsByTagName("head")[0];
HtmlElement scriptEl = _windowManager.ActiveBrowser.Document.CreateElement("script");
IHTMLScriptElement element = (IHTMLScriptElement)scriptEl.DomElement;
element.text = "window.onload = function() { document.forms[0].submit(); }";
head.AppendChild(scriptEl);
strAdditionalHeader = "";
_windowManager.ActiveBrowser.Document.InvokeScript("webBrowserControl");
To hide the softkeyboard at the time of New Activity start or onCreate(),onStart() method etc. use the code below:
getActivity().getWindow().setSoftInputMode(WindowManager.
LayoutParams.SOFT_INPUT_STATE_HIDDEN);
To hide softkeyboard at the time of Button is click in activity:
View view = this.getCurrentFocus();
if (view != null) {
InputMethodManager imm = (InputMethodManager)getSystemService(Context.INPUT_METHOD_SERVICE);
assert imm != null;
imm.hideSoftInputFromWindow(view.getWindowToken(), 0);
}
This function is good for me:
public static int getDaysCount(Date begin, Date end) {
Calendar start = org.apache.commons.lang.time.DateUtils.toCalendar(begin);
start.set(Calendar.MILLISECOND, 0);
start.set(Calendar.SECOND, 0);
start.set(Calendar.MINUTE, 0);
start.set(Calendar.HOUR_OF_DAY, 0);
Calendar finish = org.apache.commons.lang.time.DateUtils.toCalendar(end);
finish.set(Calendar.MILLISECOND, 999);
finish.set(Calendar.SECOND, 59);
finish.set(Calendar.MINUTE, 59);
finish.set(Calendar.HOUR_OF_DAY, 23);
long delta = finish.getTimeInMillis() - start.getTimeInMillis();
return (int) Math.ceil(delta / (1000.0 * 60 * 60 * 24));
}
myList = [i.split('\t')[0] for i in myList]
@echo off Title Writing using Batch Files color 0a
echo Example Text > Filename.txt echo Additional Text >> Filename.txt
@ECHO OFF
Title Writing Using Batch Files
color 0a
echo Example Text > Filename.txt
echo Additional Text >> Filename.txt
Id advice you to use a bit simplier method -
$.post('edit.php', {title: $('input[name="title"]').val() }, function(resp){
alert(resp);
});
try this one, I just feels its syntax is simplier than the $.ajax's one...
You can try to run the main function from the outline side bar of eclipse.
You could also use the below code to redirect to the main page (home):
{ path: '', redirectTo: 'home', pathMatch: 'full'}
After specifying your redirect as above, you can redirect the other pages, for example:
{ path: 'add-new-registration', component: AddNewRegistrationComponent},
{ path: 'view-registration', component: ViewRegistrationComponent},
{ path: 'home', component: HomeComponent}
you dont insert into identity fields. You need to specify the field names and use the Values clause
insert into #tmp (AssignedTo, field2, field3) values (value, value, value)
If you use do a insert into... select field field field
it will insert the first field into that identity field and will bomb
The fundamental issue with your code is that you mix two APIs. Unfortunately online resources are not great at pointing this out, but there are two semaphore APIs on UNIX-like systems:
Looking at the code above you used semget() from the System V API and tried to post through sem_post() which comes from the POSIX API. It is not possible to mix them.
To decide which semaphore API you want you don't have so many great resources. The simple best is the "Unix Network Programming" by Stevens. The section that you probably interested in is in Vol #2.
These two APIs are surprisingly different. Both support the textbook style semaphores but there are a few good and bad points in the System V API worth mentioning:
In order to read a file chosen by the user, using a file open dialog, you can use the <input type="file">
tag. You can find information on it from MSDN. When the file is chosen you can use the FileReader API to read the contents.
function onFileLoad(elementId, event) {_x000D_
document.getElementById(elementId).innerText = event.target.result;_x000D_
}_x000D_
_x000D_
function onChooseFile(event, onLoadFileHandler) {_x000D_
if (typeof window.FileReader !== 'function')_x000D_
throw ("The file API isn't supported on this browser.");_x000D_
let input = event.target;_x000D_
if (!input)_x000D_
throw ("The browser does not properly implement the event object");_x000D_
if (!input.files)_x000D_
throw ("This browser does not support the `files` property of the file input.");_x000D_
if (!input.files[0])_x000D_
return undefined;_x000D_
let file = input.files[0];_x000D_
let fr = new FileReader();_x000D_
fr.onload = onLoadFileHandler;_x000D_
fr.readAsText(file);_x000D_
}
_x000D_
<input type='file' onchange='onChooseFile(event, onFileLoad.bind(this, "contents"))' />_x000D_
<p id="contents"></p>
_x000D_
the method modify()
that you called in the last is called in global context
if you want to override modify()
you first have to inherit A
or B
.
Maybe you're trying to do this:
In this case C
inherits A
function A() {
this.modify = function() {
alert("in A");
}
}
function B() {
this.modify = function() {
alert("in B");
}
}
C = function() {
this.modify = function() {
alert("in C");
};
C.prototype.modify(); // you can call this method where you need to call modify of the parent class
}
C.prototype = new A();
SELECT
A.P_NAME AS [INDIVIDUAL NAME],B.F_DETAIL AS [INDIVIDUAL FEATURE],C.PL_PLACE AS [INDIVIDUAL LOCATION]
FROM
[dbo].[PEOPLE] A
INNER JOIN
[dbo].[FEATURE] B ON A.P_FEATURE = B.F_ID
INNER JOIN
[dbo].[PEOPLE_LOCATION] C ON A.P_LOCATION = C.PL_ID
Related to the accepted answer and another answer down the list:
I'm confirming that nanamkim's fork of custom-err-msg works with Rails 5, and with the locale setup.
You just need to start the locale message with a caret and it shouldn't display the attribute name in the message.
A model defined as:
class Item < ApplicationRecord
validates :name, presence: true
end
with the following en.yml
:
en:
activerecord:
errors:
models:
item:
attributes:
name:
blank: "^You can't create an item without a name."
item.errors.full_messages
will display:
You can't create an item without a name
instead of the usual Name You can't create an item without a name
In your case I use: val dm = ListBuffer[String]()
and val dk = ListBuffer[Map[String,anyRef]]()
essentially the shadow is the box shape just offset behind the actual box. in order to hide portions of the shadow, you need to create additional divs and set their z-index above the shadowed box so that the shadow is not visible.
If you'd like to have extremely specific control over your shadows, build them as images and created container divs with the right amount of padding and margins.. then use the png fix to make sure the shadows render properly in all browsers
I my case the problem was a residual dump file in the database directory. That file was probably generated during a backup script test I had been working on. Once I manually deleted the file I could then drop the database.
$('#input1 option').length;
This will produce 2
.
The solution I have used is:
<svg>
<line x1="100" y1="100" x2="500" y2="100" style="stroke:black; stroke-width: 2"/>
<text x="150" y="105" style="stroke:white; stroke-width:0.6em">Hello World!</text>
<text x="150" y="105" style="fill:black">Hello World!</text>
</svg>
_x000D_
A duplicate text item is being placed, with stroke and stroke-width attributes. The stroke should match the background colour, and the stroke-width should be just big enough to create a "splodge" on which to write the actual text.
A bit of a hack and there are potential issues, but works for me!
For C++ :
I just faced the same issue and I think it is worth mentioning a problem comes up when one use both __stdcall
(or WINAPI
) and extern "C"
:
As you know extern "C"
removes the decoration so that instead of :
__declspec(dllexport) int Test(void) --> dumpbin : ?Test@@YaHXZ
you obtain a symbol name undecorated:
extern "C" __declspec(dllexport) int Test(void) --> dumpbin : Test
However the _stdcall
( = macro WINAPI, that changes the calling convention) also decorates names so that if we use both we obtain :
extern "C" __declspec(dllexport) int WINAPI Test(void) --> dumpbin : _Test@0
and the benefit of extern "C"
is lost because the symbol is decorated (with _ @bytes)
Note that this only occurs for x86 architecture because the
__stdcall
convention is ignored on x64 (msdn : on x64 architectures, by convention, arguments are passed in registers when possible, and subsequent arguments are passed on the stack.).
This is particularly tricky if you are targeting both x86 and x64 platforms.
Two solutions
Use a definition file. But this forces you to maintain the state of the def file.
the simplest way : define the macro (see msdn) :
#define EXPORT comment(linker, "/EXPORT:" __FUNCTION__ "=" __FUNCDNAME__)
and then include the following pragma in the function body:
#pragma EXPORT
Full Example :
int WINAPI Test(void)
{
#pragma EXPORT
return 1;
}
This will export the function undecorated for both x86 and x64 targets while preserving the __stdcall
convention for x86. The __declspec(dllexport)
is not required in this case.
I had the same problem due to ghost processes that didn't show up when using top in bash. This prevented the JVM to spawn more threads.
For me, it resolved when listing all java processes with jps (just execute jps
in your shell) and killed them separately using the kill -9 pid
bash command for each ghost process.
This might help in some scenarios.
Various browsers (mis)handle this in various ways. Using title (an old IE 'standard') isn't particularly appropriate, since the title attribute is a mouseover effect. The jQuery solution above (Alexis) seems on the right track, but I don't think the 'error' occurs at a point where it could be caught. I've had success by replacing at the src with itself, and then catching the error:
$('img').each(function()
{
$(this).error(function()
{
$(this).replaceWith(this.alt);
}).attr('src',$(this).prop('src'));
});
This, as in the Alexis contribution, has the benefit of removing the missing img image.
If you have multiple databases for testing and development this might help
development:
adapter: mysql2
encoding: utf8
reconnect: false
database: DBNAME
pool: 5
username: usr
password: paswd
shost: localhost
test:
adapter: mysql2
encoding: utf8
reconnect: false
database: DBNAME
pool: 5
username: usr
password: paswd
shost: localhost
production:
adapter: mysql2
encoding: utf8
reconnect: false
database: DBNAME
pool: 5
username: usr
password: paswd
shost: localhost
Simply translating the "old for loop way" into streams:
private Map<String, String> mapConfig(Map<String, Integer> input, String prefix) {
int subLength = prefix.length();
return input.entrySet().stream()
.collect(Collectors.toMap(
entry -> entry.getKey().substring(subLength),
entry -> AttributeType.GetByName(entry.getValue())));
}
For float
have a look at sys.float_info
:
>>> import sys
>>> sys.float_info
sys.floatinfo(max=1.7976931348623157e+308, max_exp=1024, max_10_exp=308, min=2.2
250738585072014e-308, min_exp=-1021, min_10_exp=-307, dig=15, mant_dig=53, epsil
on=2.2204460492503131e-16, radix=2, rounds=1)
Specifically, sys.float_info.max
:
>>> sys.float_info.max
1.7976931348623157e+308
If that's not big enough, there's always positive infinity:
>>> infinity = float("inf")
>>> infinity
inf
>>> infinity / 10000
inf
The long
type has unlimited precision, so I think you're only limited by available memory.
you can easily implement by jQuery
$('input').attr('autocomplete','off');
Use "-Dspring-boot.run.profiles=foo,local" in Intellij IDEA. It's working. Its sets 2 profiles "foo and local".
Verified with boot version "2.3.2.RELEASE" & Intellij IDEA CE 2019.3.
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
Just export the environment variables you want above the check for a non-interactive shell in ~/.bashrc.
If you got a html page like this:
<body onbeforecopy = "return false" ondragstart = "return false" onselectstart = "return false" oncontextmenu = "return false" onselect = "document.selection.empty()" oncopy = "document.selection.empty()">
There a simple way to disable all events:
document.write(document.body.innerHTML)
You got the html content and lost other things.
Change this.foo()
to module.exports.foo()
Do you mean this?
var listOfList = new List<List<int>>() {
new List<int>() { 1, 2 },
new List<int>() { 3, 4 },
new List<int>() { 5, 6 }
};
var list = new List<int> { 9, 9, 9 };
var result = list.Concat(listOfList.SelectMany(x => x));
foreach (var x in result) Console.WriteLine(x);
Results in: 9 9 9 1 2 3 4 5 6
As per https://msdn.microsoft.com/en-us/library/ms180065.aspx, "DBCC USEROPTIONS reports an isolation level of 'read committed snapshot' when the database option READ_COMMITTED_SNAPSHOT is set to ON and the transaction isolation level is set to 'read committed'. The actual isolation level is read committed."
Also in SQL Server Management Studio, in database properties under Options->Miscellaneous there is "Is Read Committed Snapshot On" option status
I had this issue and it was due to the .Net framework version. I had upgraded the build to framework 4.0 but this seemed to affect some comms dlls the application was using. I rolled back to framework 3.5 and it worked fine.
I have used a trick to handle the apostrophe special character. When replacing ' for \' you need to place four backslashes before the apostrophe.
str.replaceAll("'","\\\\'");
Use
res.sendFile()
instead of
res.render()
.
What your trying to do is send a whole file.
This worked for me.
I am not sure if this applies to the older version of chosen,but now in the current version(v1.4.1) they have a option $('#autoship_option').chosen({ allow_single_deselect:true });
This will add a 'x' icon next to the name selected.Use the 'x' to clear the 'select' feild.
PS:make sure you have 'chosen-sprite.png' in the right place as per the chosen.css so that the icons are visible.
Here is my solution if you don't want to mess with additional libraries or edit your code manually. I created this Jupyter notebook to go over all java files and comment out all the Log messages. Not perfect but it got the job done for me.
Took some working, but I thougth my solution would be something to share as it is seems elegant as well as quite fast.
SELECT h.year, h.id, h.rate
FROM (
SELECT id,
SUBSTRING_INDEX(GROUP_CONCAT(CONCAT(id, '-', year) ORDER BY rate DESC), ',' , 5) AS l
FROM h
WHERE year BETWEEN 2000 AND 2009
GROUP BY id
ORDER BY id
) AS h_temp
LEFT JOIN h ON h.id = h_temp.id
AND SUBSTRING_INDEX(h_temp.l, CONCAT(h.id, '-', h.year), 1) != h_temp.l
Note that this example is specified for the purpose of the question and can be modified quite easily for other similar purposes.
Despite this question being rather old, I had to deal with a similar warning and wanted to share what I found out.
First of all this is a warning and not an error. So there is no need to worry too much about it. Basically it means, that Tomcat does not know what to do with the source
attribute from context.
This source
attribute is set by Eclipse (or to be more specific the Eclipse Web Tools Platform) to the server.xml
file of Tomcat to match the running application to a project in workspace.
Tomcat generates a warning for every unknown markup in the server.xml
(i.e. the source
attribute) and this is the source of the warning. You can safely ignore it.
you have to open the file in append mode, which can be achieved by using the FileWriter(String fileName, boolean append)
constructor.
output = new BufferedWriter(new FileWriter(my_file_name, true));
should do the trick
I read that you have a c# background. So a good starting point might be a mixin implementation for .NET.
You might want to check out the codeplex project at http://remix.codeplex.com/
Watch the lang.net Symposium link to get an overview. There is still more to come on documentation on codeplex page.
regards Stefan
char s[] = "hello";
declares s
to be an array of char
which is long enough to hold the initializer (5 + 1 char
s) and initializes the array by copying the members of the given string literal into the array.
char *s = "hello";
declares s
to be a pointer to one or more (in this case more) char
s and points it directly at a fixed (read-only) location containing the literal "hello"
.
Oracle XE With Application Express.
you just change import android.support.v7.app.ActionBarActivity; to import android.support.v7.app.AppCompatActivity;
and extends AppCompatActivity
The lowdown is: There is no solution. Excel 2011/Mac cannot correctly interpret a CSV file containing umlauts and diacritical marks no matter what encoding or hoop jumping you do. I'd be glad to hear someone tell me different!
I had the same issue, i realized i had deleted the default image that was in the folder just update the media missing, on the specific file
So, let's say you have this table:
CREATE TABLE YourTable(Col1 VARCHAR(10))
And you want to change Col1
to VARCHAR(20)
. What you need to do is this:
ALTER TABLE YourTable
ALTER COLUMN Col1 VARCHAR(20)
That'll work without problems since the length of the column got bigger. If you wanted to change it to VARCHAR(5)
, then you'll first gonna need to make sure that there are not values with more chars on your column, otherwise that ALTER TABLE
will fail.
UPDATE
The bookmarklet hack below is broken due to XHR issues and API changes.
Thankfully Github now has "A Whole New Code Search" which does the job superbly.
Checkout this voodoo: Github code search userscript.
Follow the directions there, or if you hate bloating your browser with scripts and extensions, use my bookmarkified bundle of the userscript:
javascript:(function(){var s='https://raw.githubusercontent.com/skratchdot/github-enhancement-suite/master/build/github-enhancement-suite.user.js',t='text/javascript',d=document,n=navigator,e;(e=d.createElement('script')).src=s;e.type=t;d.getElementsByTagName('head')[0].appendChild(e)})();doIt('');void('');
Save the source above as the URL of a new bookmark. Browse to any Github repo, click the bookmark, and bam: in-page, ajaxified code search.
CAVEAT Github must index a repo before you can search it.
Abracadabra...
Here's a sample search from the annotated ECMAScript 5.1 specification repository:
In case you have a loop with an async request in each one and you want a certain time between each request you can use this code:
var startTimeout = function(timeout, i){
setTimeout(function() {
myAsyncFunc(i).then(function(data){
console.log(data);
})
}, timeout);
}
var myFunc = function(){
timeout = 0;
i = 0;
while(i < 10){
// By calling a function, the i-value is going to be 1.. 10 and not always 10
startTimeout(timeout, i);
// Increase timeout by 1 sec after each call
timeout += 1000;
i++;
}
}
This examples waits 1 second after each request before sending the next one.
//to get day of any date
import java.util.Scanner;
import java.util.Calendar;
import java.util.Date;
public class Show {
public static String getDay(String day,String month, String year){
String input_date = month+"/"+day+"/"+year;
Date now = new Date(input_date);
Calendar calendar = Calendar.getInstance();
calendar.setTime(now);
int final_day = (calendar.get(Calendar.DAY_OF_WEEK));
String finalDay[]={"SUNDAY","MONDAY","TUESDAY","WEDNESDAY","THURSDAY","FRIDAY","SATURDAY"};
System.out.println(finalDay[final_day-1]);
}
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
String month = in.next();
String day = in.next();
String year = in.next();
getDay(day, month, year);
}
}
Just add in the '0' in your select.
INSERT INTO table_name (a,b,c,d)
SELECT
other_table.a AS a,
other_table.b AS b,
other_table.c AS c,
'0' AS d
FROM other_table
While there are many ways to skin this cat, I prefer to wrap such code into reusable extension methods that make it trivial to do going forward. When using extension methods, you can also avoid RegEx as it is slower than a direct character check. I like using the extensions in the Extensions.cs NuGet package. It makes this check as simple as:
using Extensions;
" to the top of your code."smith23".IsAlphaNumeric()
will return True whereas "smith 23".IsAlphaNumeric(false)
will return False. By default the .IsAlphaNumeric()
method ignores spaces, but it can also be overridden as shown above. If you want to allow spaces such that "smith 23".IsAlphaNumeric()
will return True, simple default the arg.MyString.IsAlphaNumeric()
.March 2014: Good news! Python 3.4 ships with Pip. Pip has long been Python's de-facto standard package manager. You can install a package like this:
pip install httpie
Wahey! This is the best feature of any Python release. It makes the community's wealth of libraries accessible to everyone. Newbies are no longer excluded from using community libraries by the prohibitive difficulty of setup.
However, there remains a number of outstanding frustrations with the Python packaging experience. Cumulatively, they make Python very unwelcoming for newbies. Also, the long history of neglect (ie. not shipping with a package manager for 14 years from Python 2.0 to Python 3.3) did damage to the community. I describe both below.
It's important to understand that while experienced users are able to work around these frustrations, they are significant barriers to people new to Python. In fact, the difficulty and general user-unfriendliness is likely to deter many of them.
Every language with a package manager has an official (or quasi-official) repository for the community to download and publish packages. Python has the Python Package Index, PyPI. https://pypi.python.org/pypi
Let's compare its pages with those of RubyGems and Npm (the Node package manager).
rails
express
simplejson
You'll see the RubyGems and Npm pages both begin with a one-line description of the package, then large friendly instructions how to install it.
Meanwhile, woe to any hapless Python user who naively browses to PyPI. On https://pypi.python.org/pypi/simplejson/ , they'll find no such helpful instructions. There is however, a large green 'Download' link. It's not unreasonable to follow it. Aha, they click! Their browser downloads a .tar.gz
file. Many Windows users can't even open it, but if they persevere they may eventually extract it, then run setup.py
and eventually with the help of Google setup.py install
. Some will give up and reinvent the wheel..
Of course, all of this is wrong. The easiest way to install a package is with a Pip command. But PyPI didn't even mention Pip. Instead, it led them down an archaic and tedious path.
Numpy is one of Python's most popular libraries. Try to install it with Pip, you get this cryptic error message:
Error: Unable to find vcvarsall.bat
Trying to fix that is one of the most popular questions on Stack Overflow: "error: Unable to find vcvarsall.bat"
Few people succeed.
For comparison, in the same situation, Ruby prints this message, which explains what's going on and how to fix it:
Please update your PATH to include build tools or download the DevKit from http://rubyinstaller.org/downloads and follow the instructions at http://github.com/oneclick/rubyinstaller/wiki/Development-Kit
Ruby and Nodejs ship with full-featured package managers, Gem (since 2007) and Npm (since 2011), and have nurtured sharing communities centred around GitHub. Npm makes publishing packages as easy as installing them, it already has 64k packages. RubyGems lists 72k packages. The venerable Python package index lists only 41k.
Flying in the face of its "batteries included" motto, Python shipped without a package manager until 2014.
Until Pip, the de facto standard was a command easy_install
. It was woefully inadequate. The was no command to uninstall packages.
Pip was a massive improvement. It had most the features of Ruby's Gem. Unfortunately, Pip was--until recently--ironically difficult to install. In fact, the problem remains a top Python question on Stack Overflow: "How do I install pip on Windows?"
I have solved this problem in my pycharm in a bit different way.
Go to settings -> Project Interpreter and then click on the base package there.
You will see a page like this
After that when your package is installed then you should see the package is colored blue rather than white.
And the unresolved reference is also gone too.
as per docs:
"json"
: Evaluates the response as JSON and returns a JavaScript object. In jQuery 1.4 the JSON data is parsed in a strict manner; any malformed JSON is rejected and a parse error is thrown. (See json.org for more information on proper JSON formatting.)"text"
: A plain text string.Divs stack vertically by default, so there is no need for special handling of "rows" within a column.
div {_x000D_
height:50px;_x000D_
}_x000D_
.short-div {_x000D_
height:25px;_x000D_
}
_x000D_
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" rel="stylesheet" />_x000D_
_x000D_
<div class="container">_x000D_
<h1>Responsive Bootstrap</h1>_x000D_
<div class="row">_x000D_
<div class="col-lg-5 col-md-5 col-sm-5 col-xs-5" style="background-color:red;">Span 5</div>_x000D_
<div class="col-lg-3 col-md-3 col-sm-3 col-xs-3" style="background-color:blue">Span 3</div>_x000D_
<div class="col-lg-2 col-md-2 col-sm-3 col-xs-2" style="padding:0px">_x000D_
<div class="short-div" style="background-color:green">Span 2</div>_x000D_
<div class="short-div" style="background-color:purple">Span 2</div>_x000D_
</div>_x000D_
<div class="col-lg-2 col-md-2 col-sm-3 col-xs-2" style="background-color:yellow">Span 2</div>_x000D_
</div>_x000D_
</div>_x000D_
<div class="container-fluid">_x000D_
<div class="row-fluid">_x000D_
<div class="col-lg-6 col-md-6 col-sm-6 col-xs-6">_x000D_
<div class="short-div" style="background-color:#999">Span 6</div>_x000D_
<div class="short-div">Span 6</div>_x000D_
</div>_x000D_
<div class="col-lg-6 col-md-6 col-sm-6 col-xs-6" style="background-color:#ccc">Span 6</div>_x000D_
</div>_x000D_
</div>
_x000D_
Here's the fiddle.
For a terse, pure flexbox option, group the left-aligned items and the right-aligned items:
<div class="wrap">
<div>
<span>One</span>
<span>Two</span>
</div>
<div>Three</div>
</div>
and use space-between
:
.wrap {
display: flex;
background: #ccc;
justify-content: space-between;
}
This way you can group multiple items to the right(or just one).
UPDATE:
You'll also want to goto Security -> Server Roles
, and for public
check the permissions for TSQL Default TCP/TSQL Default VIA/TSQL Local Machine/TSQL Named Pipes
and remove the connect
permission
To start with Socket.IO I suggest you read first the example on the main page:
On the server side, read the "How to use" on the GitHub source page:
https://github.com/Automattic/socket.io
And on the client side:
https://github.com/Automattic/socket.io-client
Finally you need to read this great tutorial:
http://howtonode.org/websockets-socketio
Hint: At the end of this blog post, you will have some links pointing on source code that could be some help.
class Second:
def __init__(self, data):
self.data = data
class First:
def SecondClass(self, data):
return Second(data)
FirstClass = First()
SecondClass = FirstClass.SecondClass('now you see me')
print SecondClass.data
It looks it's essential to call decode() function to make use of actual string data even after calling base64.b64decode over base64 encoded string. Because never forget it always return bytes literals.
import base64
conv_bytes = bytes('your string', 'utf-8')
print(conv_bytes) # b'your string'
encoded_str = base64.b64encode(conv_bytes)
print(encoded_str) # b'eW91ciBzdHJpbmc='
print(base64.b64decode(encoded_str)) # b'your string'
print(base64.b64decode(encoded_str).decode()) # your string
I tried almost all of these suggestions, but they were all returning empty values. If anyone else has this issue, I found this handy function on php.net (http://php.net/manual/en/function.get-current-user.php):
get_current_user();
$username = get_current_user();
echo $username;
This was the only way I was finally able to get the user's active directory username. If none of the above answers has worked, give this a try.
double[] array = new double[1048576];
...
By default order is ascending
To reverse the order
Arrays.sort(array,Collections.reverseOrder());
If this is your problem, you should probably implement another way to do the view filling in your Activity. Instead of re running onCreate()
you should make it so onCreate()
calls your filling method with some argument. When the data changes, the filling method should get called with another argument.
If you want a specific order, then you must use an array, not an object. Objects do not have a defined order.
For example, using an array, you could do this:
var myobj = [{"A":["B"]}, {"B": ["C"]}];
var firstItem = myobj[0];
Then, you can use myobj[0] to get the first object in the array.
Or, depending upon what you're trying to do:
var myobj = [{key: "A", val:["B"]}, {key: "B", val:["C"]}];
var firstKey = myobj[0].key; // "A"
var firstValue = myobj[0].val; // "["B"]
RequireJS implements the AMD API (source).
CommonJS is a way of defining modules with the help of an exports
object, that defines the module contents. Simply put, a CommonJS implementation might work like this:
// someModule.js
exports.doSomething = function() { return "foo"; };
//otherModule.js
var someModule = require('someModule'); // in the vein of node
exports.doSomethingElse = function() { return someModule.doSomething() + "bar"; };
Basically, CommonJS specifies that you need to have a require()
function to fetch dependencies, an exports
variable to export module contents and a module identifier (which describes the location of the module in question in relation to this module) that is used to require the dependencies (source). CommonJS has various implementations, including Node.js, which you mentioned.
CommonJS was not particularly designed with browsers in mind, so it doesn't fit in the browser environment very well (I really have no source for this--it just says so everywhere, including the RequireJS site.) Apparently, this has something to do with asynchronous loading, etc.
On the other hand, RequireJS implements AMD, which is designed to suit the browser environment (source). Apparently, AMD started as a spinoff of the CommonJS Transport format and evolved into its own module definition API. Hence the similarities between the two. The new feature in AMD is the define()
function that allows the module to declare its dependencies before being loaded. For example, the definition could be:
define('module/id/string', ['module', 'dependency', 'array'],
function(module, factory function) {
return ModuleContents;
});
So, CommonJS and AMD are JavaScript module definition APIs that have different implementations, but both come from the same origins.
To confuse you even more, RequireJS, while being an AMD implementation, offers a CommonJS wrapper so CommonJS modules can almost directly be imported for use with RequireJS.
define(function(require, exports, module) {
var someModule = require('someModule'); // in the vein of node
exports.doSomethingElse = function() { return someModule.doSomething() + "bar"; };
});
I hope this helps to clarify things!
If you're able to use Underscore.js in your project, the _.filter() array function makes this a snap:
// find all strings in array containing 'thi'
var matches = _.filter(
[ 'item 1', 'thing', 'id-3-text', 'class' ],
function( s ) { return s.indexOf( 'thi' ) !== -1; }
);
The iterator function can do whatever you want as long as it returns true for matches. Works great.
Update 2017-12-03:
This is a pretty outdated answer now. Maybe not the most performant option in a large batch, but it can be written a lot more tersely and use native ES6 Array/String methods like .filter()
and .includes()
now:
// find all strings in array containing 'thi'
const items = ['item 1', 'thing', 'id-3-text', 'class'];
const matches = items.filter(s => s.includes('thi'));
Note: There's no <= IE11 support for String.prototype.includes()
(Edge works, mind you), but you're fine with a polyfill, or just fall back to indexOf()
.
The 3-Heights™ PDF Validator Online Tool provides good feedback for different PDF/A conformance levels and versions.
This structure (function() {})();
is called IIFE (Immediately Invoked Function Expression), it will be executed immediately, when the interpreter will reach this line. So when you're writing these rows:
(function($) {
// do something
})(jQuery);
this means, that the interpreter will invoke the function immediately, and will pass jQuery
as a parameter, which will be used inside the function as $
.
This is the best way to enable/ disable swipe to pop view controller in iOS 10, Swift 3 :
For First Screen [ Where you want to Disable Swipe gesture ] :
class SignUpViewController : UIViewController,UIGestureRecognizerDelegate {
//MARK: - View initializers
override func viewDidLoad() {
super.viewDidLoad()
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
swipeToPop()
}
override func viewWillDisappear(_ animated: Bool) {
super.viewWillDisappear(animated)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
func swipeToPop() {
self.navigationController?.interactivePopGestureRecognizer?.isEnabled = true;
self.navigationController?.interactivePopGestureRecognizer?.delegate = self;
}
func gestureRecognizerShouldBegin(_ gestureRecognizer: UIGestureRecognizer) -> Bool {
if gestureRecognizer == self.navigationController?.interactivePopGestureRecognizer {
return false
}
return true
} }
For middle screen [ Where you want to Enable Swipe gesture ] :
class FriendListViewController : UIViewController {
//MARK: - View initializers
override func viewDidLoad() {
super.viewDidLoad()
swipeToPop()
}
func swipeToPop() {
self.navigationController?.interactivePopGestureRecognizer?.isEnabled = true;
self.navigationController?.interactivePopGestureRecognizer?.delegate = nil;
} }
Right Click on your project: Run (As) -> Maven clean Right Click on your project: Run (As) -> Maven install
I'm not familiar with Wordpress templates, but I'm assuming that headers are sent to the browser by WP before your template is even loaded. Because of that, the common redirection method of:
header("Location: new_url");
won't work. Unless there's a way to force sending headers through a template before WP does anything, you'll need to use some Javascript like so:
<script language="javascript" type="text/javascript">
document.location = "new_url";
</script>
Put that in the section and it'll be run when the page loads. This method won't be instant, and it also won't work for people with Javascript disabled.
if (!obj) {
// object (not class!) doesn't exist yet
}
else ...
This is what I did:
EF:
using (var context = new SomeDBContext())
{
foreach (var item in model.ShopItems) // ShopItems is a posted list with values
{
var feature = context.Shop
.Where(h => h.ShopID == 123 && h.Type == item.Type).ToList();
feature.ForEach(a => a.SortOrder = item.SortOrder);
}
context.SaveChanges();
}
Hope helps someone.
Nope, you have to open a browser atleast once to create your username
on GitHub, once created, you can leverage GitHub API to create repositories from command line, following below command:
curl -u 'github-username' https://api.github.com/user/repos -d '{"name":"repo-name"}'
For example:
curl -u 'arpitaggarwal' https://api.github.com/user/repos -d '{"name":"command-line-repo"}'
Wildcard method: Add the following entry into your DNS server and change the domain and IP address accordingly.
*.example.com IN A 1.2.3.4
The correct way of referring to a method parameter is like this:
From the terminal (osx) I usually use
cordova plugin -l | xargs cordova plugins rm
Pipe, pipe everything!
To expand a bit: this command will loop through the results of cordova plugin -l
and feed it to cordova plugins rm
.
xargs is one of those commands that you wonder why you didn't know about before. See this tut.
From the Markdown Cheatsheet:
You can add it to your repo and reference it with an image tag:
Inline-style:

Reference-style:
![alt text][logo]
[logo]: https://github.com/adam-p/markdown-here/raw/master/src/common/images/icon48.png "Logo Title Text 2"
Inline-style:
Reference-style:
Alternatively you can use the url directly:
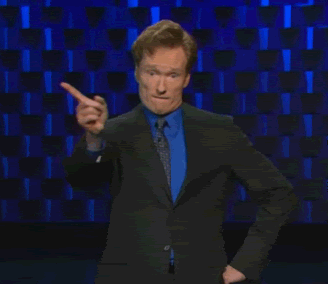
Select the commit you would like to roll back to and reverse the changes by clicking Reverse File
, Reverse Hunk
or Reverse Selected Lines
. Do this for all the commits after the commit you would like to roll back to also.
Right click on the commit and click on Reset current branch to this commit
.
Thing is, the code you provided won't work because the <iframe>
element has to have a "src" property, like:
<iframe id="uploads" src="http://domain/page.html"></iframe>
It's ok to use .contents()
to get the content:
$('#uploads).contents()
will give you access to the second iframe, but if that iframe is "INSIDE" the http://domain/page.html
document the #uploads iframe loaded.
To test I'm right about this, I created 3 html files named main.html, iframe.html and noframe.html and then selected the div#element just fine with:
$('#uploads').contents().find('iframe').contents().find('#element');
There WILL be a delay in which the element will not be available since you need to wait for the iframe to load the resource. Also, all iframes have to be on the same domain.
Hope this helps ...
Here goes the html for the 3 files I used (replace the "src" attributes with your domain and url):
main.html
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<title>main.html example</title>
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<script>
$(function () {
console.log( $('#uploads').contents().find('iframe').contents().find('#element') ); // nothing at first
setTimeout( function () {
console.log( $('#uploads').contents().find('iframe').contents().find('#element') ); // wait and you'll have it
}, 2000 );
});
</script>
</head>
<body>
<iframe id="uploads" src="http://192.168.1.70/test/iframe.html"></iframe>
</body>
iframe.html
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<title>iframe.html example</title>
</head>
<body>
<iframe src="http://192.168.1.70/test/noframe.html"></iframe>
</body>
noframe.html
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<title>noframe.html example</title>
</head>
<body>
<div id="element">some content</div>
</body>
If you will never need to serialize your objects to byte array and send/store them, then you don't need to worry about it. If you do, then you must consider your serialVersionUID since the deserializer of the object will match it to the version of object its classloader has. Read more about it in the Java Language Specification.
You would have used inline styles at many places, which CSP(Content Security Policy) prohibits because it could be dangerous.
Just try removing those inline styles and put it inside dedicated stylesheet.
According to http://php.net/manual/en/function.error-get-last.php, use:
print_r(error_get_last());
Which will return an array of the last error generated. You can access the [message]
element to display the error.
I had the same problem but changeing max_allowed_packet in the my.ini/my.cnf file under [mysqld] made the trick.
add a line
max_allowed_packet=500M
now restart the MySQL service once you are done.
My version, in my humble opinion, more readable:
SELECT PARENT.TABLE_NAME "PARENT TABLE_NAME"
, PARENT.CONSTRAINT_NAME "PARENT PK CONSTRAINT"
, '->' " "
, CHILD.TABLE_NAME "CHILD TABLE_NAME"
, CHILD.COLUMN_NAME "CHILD COLUMN_NAME"
, CHILD.CONSTRAINT_NAME "CHILD CONSTRAINT_NAME"
FROM ALL_CONS_COLUMNS CHILD
, ALL_CONSTRAINTS CT
, ALL_CONSTRAINTS PARENT
WHERE CHILD.OWNER = CT.OWNER
AND CT.CONSTRAINT_TYPE = 'R'
AND CHILD.CONSTRAINT_NAME = CT.CONSTRAINT_NAME
AND CT.R_OWNER = PARENT.OWNER
AND CT.R_CONSTRAINT_NAME = PARENT.CONSTRAINT_NAME
AND CHILD.TABLE_NAME = ::table -- table name variable
AND CT.OWNER = ::owner; -- schema variable, could not be needed
Delete these 3 files present in your local at path C:\ProgramData\Oracle\Java\javapath
java.exe
javaw.exe
javaws.exe
This solved the issue for me :)
column_obj != None
will produce a IS NOT NULL
constraint:
In a column context, produces the clause
a != b
. If the target isNone
, produces aIS NOT NULL
.
or use isnot()
(new in 0.7.9):
Implement the
IS NOT
operator.Normally,
IS NOT
is generated automatically when comparing to a value ofNone
, which resolves toNULL
. However, explicit usage ofIS NOT
may be desirable if comparing to boolean values on certain platforms.
Demo:
>>> from sqlalchemy.sql import column
>>> column('YourColumn') != None
<sqlalchemy.sql.elements.BinaryExpression object at 0x10c8d8b90>
>>> str(column('YourColumn') != None)
'"YourColumn" IS NOT NULL'
>>> column('YourColumn').isnot(None)
<sqlalchemy.sql.elements.BinaryExpression object at 0x104603850>
>>> str(column('YourColumn').isnot(None))
'"YourColumn" IS NOT NULL'
In addition to the answer, $ yarn cache clean
removes all libraries from cache. If you want to remove a specific lib's cache run $ yarn cache dir
to get the right yarn cache directory path for your OS, then $ cd
to that directory and remove the folder with the name
+ version
of the lib you want to cleanup.
In the constructor method of your model class set the default value whatever you want. Then in your first action create an instance of the model and pass it to your view.
public ActionResult VolunteersAdd()
{
VolunteerModel model = new VolunteerModel(); //to set the default values
return View(model);
}
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult VolunteersAdd(VolunteerModel model)
{
return View(model);
}
You need to set basicHttpBinding -> MaxReceivedMessageSize in the client configuration.
function showStuff(id, text, btn) {_x000D_
document.getElementById(id).style.display = 'block';_x000D_
// hide the lorem ipsum text_x000D_
document.getElementById(text).style.display = 'none';_x000D_
// hide the link_x000D_
btn.style.display = 'none';_x000D_
}
_x000D_
<td class="post">_x000D_
_x000D_
<a href="#" onclick="showStuff('answer1', 'text1', this); return false;">Edit</a>_x000D_
<span id="answer1" style="display: none;">_x000D_
<textarea rows="10" cols="115"></textarea>_x000D_
</span>_x000D_
_x000D_
<span id="text1">Lorem ipsum Lorem ipsum Lorem ipsum Lorem ipsum</span>_x000D_
</td>
_x000D_
Or, you can use a control
class instead of their types:
GridViewRow row = (GridViewRow)(((Control)e.CommandSource).NamingContainer);
int RowIndex = row.RowIndex;
Here's my twist on it, with a runnable example. Note this will only work in the situation where Id
is unique, and you have duplicate values in other columns.
DECLARE @SampleData AS TABLE (Id int, Duplicate varchar(20))
INSERT INTO @SampleData
SELECT 1, 'ABC' UNION ALL
SELECT 2, 'ABC' UNION ALL
SELECT 3, 'LMN' UNION ALL
SELECT 4, 'XYZ' UNION ALL
SELECT 5, 'XYZ'
DELETE FROM @SampleData WHERE Id IN (
SELECT Id FROM (
SELECT
Id
,ROW_NUMBER() OVER (PARTITION BY [Duplicate] ORDER BY Id) AS [ItemNumber]
-- Change the partition columns to include the ones that make the row distinct
FROM
@SampleData
) a WHERE ItemNumber > 1 -- Keep only the first unique item
)
SELECT * FROM @SampleData
And the results:
Id Duplicate
----------- ---------
1 ABC
3 LMN
4 XYZ
Not sure why that's what I thought of first... definitely not the simplest way to go but it works.
First you should check if books
itself isn't null, then simply check whether books[i] != null
:
if(books==null) throw new IllegalArgumentException();
for (int i = 0; i < books.length; i++){
if(books[i] != null){
total += books[i].getPrice();
}
}
There are three ways of handling business logic in AngularJS: (Inspired by Yaakov's Coursera AngularJS course) which are:
Here we are only going to talk about Service vs Factory
SERVICE:
Syntax:
app.js
var app = angular.module('ServiceExample',[]);
var serviceExampleController =
app.controller('ServiceExampleController', ServiceExampleController);
var serviceExample = app.service('NameOfTheService', NameOfTheService);
ServiceExampleController.$inject = ['NameOfTheService'] //very important as this protects from minification of js files
function ServiceExampleController(NameOfTheService){
serviceExampleController = this;
serviceExampleController.data = NameOfTheService.getSomeData();
}
function NameOfTheService(){
nameOfTheService = this;
nameOfTheService.data = "Some Data";
nameOfTheService.getSomeData = function(){
return nameOfTheService.data;
}
}
index.html
<div ng-controller = "ServiceExampleController as serviceExample">
{{serviceExample.data}}
</div>
The main features of Service:
Lazily Instantiated: If the service is not injected it won't be instantiated ever. So to use it you will have to inject it to a module.
Singleton: If it is injected to multiple modules, all will have access to only one particular instance. That is why, it is very convenient to share data across different controllers.
FACTORY
Now let's talk about the Factory in AngularJS
First let's have a look at the syntax:
app.js:
var app = angular.module('FactoryExample',[]);
var factoryController = app.controller('FactoryController', FactoryController);
var factoryExampleOne = app.factory('NameOfTheFactoryOne', NameOfTheFactoryOne);
var factoryExampleTwo = app.factory('NameOfTheFactoryTwo', NameOfTheFactoryTwo);
//first implementation where it returns a function
function NameOfTheFactoryOne(){
var factory = function(){
return new SomeService();
}
return factory;
}
//second implementation where an object literal would be returned
function NameOfTheFactoryTwo(){
var factory = {
getSomeService : function(){
return new SomeService();
}
};
return factory;
}
Now using the above two in the controller:
var factoryOne = NameOfTheFactoryOne() //since it returns a function
factoryOne.someMethod();
var factoryTwo = NameOfTheFactoryTwo.getSomeService(); //accessing the object
factoryTwo.someMethod();
Features of Factory:
This types of services follow the factory design pattern. The factory can be thought of as a central place that creates new objects or methods.
This does not only produce singleton, but also customizable services.
The .service()
method is a factory that always produces the same type of service, which is a singleton. There is no easy way to configure it's behavior. That .service()
method is usually used as a shortcut for something that doesn't require any configuration whatsoever.
My 2 cents:
using (ZipArchive archive = ZipFile.Open(zFile, ZipArchiveMode.Create))
{
foreach (var fPath in filePaths)
{
archive.CreateEntryFromFile(fPath,Path.GetFileName(fPath));
}
}
So Zip files could be created directly from files/dirs.
You need to declare out
as a byte array with a length equal to the lengths of ciphertext
and mac
added together, and then copy ciphertext
over the beginning of out
and mac
over the end, using arraycopy.
byte[] concatenateByteArrays(byte[] a, byte[] b) {
byte[] result = new byte[a.length + b.length];
System.arraycopy(a, 0, result, 0, a.length);
System.arraycopy(b, 0, result, a.length, b.length);
return result;
}
I think there is little difference between the two events. To understand this, I created a simple example to manipulation:
XAML
<Window x:Class="LoadedAndContentRendered.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Name="MyWindow"
Title="MainWindow" Height="1000" Width="525"
WindowStartupLocation="CenterScreen"
ContentRendered="Window_ContentRendered"
Loaded="Window_Loaded">
<Grid Name="RootGrid">
</Grid>
</Window>
Code behind
private void Window_ContentRendered(object sender, EventArgs e)
{
MessageBox.Show("ContentRendered");
}
private void Window_Loaded(object sender, RoutedEventArgs e)
{
MessageBox.Show("Loaded");
}
In this case the message Loaded
appears the first after the message ContentRendered
. This confirms the information in the documentation.
In general, in WPF the Loaded
event fires if the element:
is laid out, rendered, and ready for interaction.
Since in WPF the Window
is the same element, but it should be generally content that is arranged in a root panel (for example: Grid
). Therefore, to monitor the content of the Window
and created an ContentRendered
event. Remarks from MSDN:
If the window has no content, this event is not raised.
That is, if we create a Window
:
<Window x:Class="LoadedAndContentRendered.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Name="MyWindow"
ContentRendered="Window_ContentRendered"
Loaded="Window_Loaded" />
It will only works Loaded
event.
With regard to access to the elements in the Window
, they work the same way. Let's create a Label
in the main Grid
of Window
. In both cases we have successfully received access to Width
:
private void Window_ContentRendered(object sender, EventArgs e)
{
MessageBox.Show("ContentRendered: " + SampleLabel.Width.ToString());
}
private void Window_Loaded(object sender, RoutedEventArgs e)
{
MessageBox.Show("Loaded: " + SampleLabel.Width.ToString());
}
As for the Styles
and Templates
, at this stage they are successfully applied, and in these events we will be able to access them.
For example, we want to add a Button
:
private void Window_ContentRendered(object sender, EventArgs e)
{
MessageBox.Show("ContentRendered: " + SampleLabel.Width.ToString());
Button b1 = new Button();
b1.Content = "ContentRendered Button";
RootGrid.Children.Add(b1);
b1.Height = 25;
b1.Width = 200;
b1.HorizontalAlignment = HorizontalAlignment.Right;
}
private void Window_Loaded(object sender, RoutedEventArgs e)
{
MessageBox.Show("Loaded: " + SampleLabel.Width.ToString());
Button b1 = new Button();
b1.Content = "Loaded Button";
RootGrid.Children.Add(b1);
b1.Height = 25;
b1.Width = 200;
b1.HorizontalAlignment = HorizontalAlignment.Left;
}
In the case of Loaded
event, Button
to add to Grid
immediately at the appearance of the Window
. In the case of ContentRendered
event, Button
to add to Grid
after all its content will appear.
Therefore, if you want to add items or changes before load Window
you must use the Loaded
event. If you want to do the operations associated with the content of Window
such as taking screenshots you will need to use an event ContentRendered
.
Use typings to access node functions from TypeScript:
typings install env~node --global
If you don't have typings install it:
npm install typings --global
Just for the sake of completeness: there are IPv4-mapped IPv6 addresses, where you can embed an IPv4 address in an IPv6 address (may not be supported by every IPv6 equipment).
Example: I run a server on my machine, which can be accessed via http://127.0.0.1:19983/solr
. If I access it via an IPv4-mapped IPv6 address then I access it via http://[::ffff:127.0.0.1]:19983/solr
(which will be converted to http://[::ffff:7f00:1]:19983/solr
)
There are MD5 libraries available for javascript. Keep in mind that this solution will not work if you need to support users who do not have javascript available.
The more common solution is to use HTTPS. With HTTPS, SSL encryption is negotiated between your web server and the client, transparently encrypting all traffic.
As a web user, you can export by going to Job Config History, then exporting XML.
I'm in the situation of not having access to the machine Jenkins is running on and wanted to export as a backup.
As for importing the xml as a web user, I'd still like to know.
if the page have one <a>
It Works,but,many <a>
,have to use var href = $(this).attr('href');
Just use it...
Firstly include the unistd.h
header file, #include<unistd.h>
, and use this function for pausing your program execution for desired number of seconds:
sleep(x);
x
can take any value in seconds.
If you want to pause the program for 5 seconds it is like this:
sleep(5);
It is correct and I use it frequently.
It is valid for C and C++.
All of the snippets on this page can be dramatically reduced in size.
The mysqli result set object can be immediately fed to a foreach()
(because it is "iterable") which eliminates the need to maked iterated _fetch()
calls.
Imploding each row will allow your code to correctly print all columnar data in the result set without modifying the code.
$sql = "SELECT * FROM MY_TABLE";
echo '<table>';
foreach (mysqli_query($conn, $sql) as $row) {
echo '<tr><td>' . implode('</td><td>', $row) . '</td></tr>';
}
echo '</table>';
If you want to encode html entities, you can map each row:
implode('</td><td>' . array_map('htmlspecialchars', $row))
If you don't want to use implode, you can simply access all row data using associative array syntax. ($row['id']
)
Put the part from BEGIN....END{}
inside a file and name it like my.awk.
And then execute it like below:
awk -f my.awk life.csv >output.txt
Also I see a field separator as ,
. You can add that in the begin block of the .awk file as FS=","
I'm in windows 10, using WAMP64 server. Searched for my.cnf
and my.ini
. Found my.ini
in C:\wamp64\bin\mariadb\mariadb10.2.14
.
Following the instructions from the colleagues:
my.ini
in a text editor, searched for [mysqld]
'skip-grant-tables'
at the end of the [mysqld]
section (but within it)skip-grant-tables
optionALTER USER 'root'@'localhost' IDENTIFIED WITH mysql_native_password BY 'newpassword';
my.ini
file, erase the 'skip-grant-tables'
line, save the fileNow you can enter with the new password. Thanks to all answers here.
1) Try putting an absolute link not relative link to your eot font - somehow old IE just don't know in which folder the css file is 2) make 2 extra @font-face declarations so it should look like this:
@font-face { /* for modern browsers and modern IE */
font-family: "Futura";
src: url("../fonts/Futura_Medium_BT.eot");
src: url("../fonts/Futura_Medium_BT.eot?#iefix") format("embedded-opentype"),
url( "../fonts/Futura_Medium_BT.ttf" ) format("truetype");
}
@font-face{ /* for old IE */
font-family: "Futura_IE";
src: url(/wp-content/themes/my-theme/fonts/Futura_Medium_BT.eot);
}
@font-face{ /* for old IE */
font-family: "Futura_IE2";
src:url(/wp-content/themes/my-theme/fonts/Futura_Medium_BT.eot?#iefix)
format("embedded-opentype");
}
.p{ font-family: "Futura", "Futura_IE", "Futura_IE2", Arial, sans-serif;
This is an example for wordpress template - absolute link should point from where your start index file is.
I have found that you must be on the latest commit of the git. So these are the steps to take: 1) make sure you have not been working on the same files, otherwise you will run into a DITY_WORK_TREE error. 2) pull the latest changes. 3) commit your updates.
Hope this helps.
How to add single item. This is not very effective but follows what you are asking for:
x = p.Series()
N = 4
for i in xrange(N):
x = x.set_value(i, i**2)
produces x:
0 0
1 1
2 4
3 9
Obviously there are better ways to generate this series in only one shot.
For your second question check answer and references of SO question add one row in a pandas.DataFrame.
I want to add some clarification to this thread:
Update-Database -TargetMigration:"name_of_migration"
What you are doing above is saying that you want to rollback all migrations UNTIL you're left with the migration specified. Thus, if you use GET-MIGRATIONS and you find that you have A, B, C, D, and E, then using this command will rollback E and D to get you to C:
Update-Database -TargetMigration:"C"
Also, unless anyone can comment to the contrary, I noticed that you can use an ordinal value and the short -Target switch (thus, -Target is the same as -TargetMigration). If you want to rollback all migrations and start over, you can use:
Update-Database -Target:0
0, above, would rollback even the FIRST migration (this is a destructive command--be sure you know what you're doing before you use it!)--something you cannot do if you use the syntax above that requires the name of the target migration (the name of the 0th migration doesn't exist before a migration is applied!). So in that case, you have to use the 0 (ordinal) value. Likewise, if you have applied migrations A, B, C, D, and E (in that order), then the ordinal 1 should refer to A, ordinal 2 should refer to B, and so on. So to rollback to B you could use either:
Update-Database -TargetMigration:"B"
or
Update-Database -TargetMigration:2
According to this related answer on a similar question, correct command is -Target
for EF Core 1.1 while it is -Migration
for EF Core 2.0.
You won't believe this, Make sure to add "." at the end of the "url"
I got a similar error with this code:
fetch(https://itunes.apple.com/search?term=jack+johnson)
.then( response => {
return response.json();
})
.then(data => {
console.log(data.results);
}).catch(error => console.log('Request failed:', error))
The error I got:
Access to fetch at 'https://itunes.apple.com/search?term=jack+johnson'
from origin 'http://127.0.0.1:5500' has been blocked by CORS policy:
No 'Access-Control-Allow-Origin' header is present on the requested
resource. If an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled.
But I realized after a lot of research that the problem was that I did not copy the right URL address from the iTunes API documentation.
It should have been
https://itunes.apple.com/search?term=jack+johnson.
not
https://itunes.apple.com/search?term=jack+johnson
Notice the dot at the end
There is a huge explanation about why the dot is important quoting issues about DNS and character encoding but the truth is you probably do not care. Try adding the dot it might work for you too.
When I added the "." everything worked like a charm.
I hope it works for you too.
Place a scroll view on your scene, and size it so that it fills the scene. Then, place a stack view inside the scroll view, and place the add item button inside the stack view. As soon as everything’s in place, set the following constraints:
Scroll View.Leading = Superview.LeadingMargin
Scroll View.Trailing = Superview.TrailingMargin
Scroll View.Top = Superview.TopMargin
Bottom Layout Guide.Top = Scroll View.Bottom + 20.0
Stack View.Leading = Scroll View.Leading
Stack View.Trailing = Scroll View.Trailing
Stack View.Top = Scroll View.Top
Stack View.Bottom = Scroll View.Bottom
Stack View.Width = Scroll View.Width
code:Stack View.Width = Scroll View.Width
is the key.
//CSS
.navigation_bar > ul > li {
list-style: none;
display: inline;
margin: 2%;
}
.link {
text-decoration: none;
}
//JSX
<div className="navigation_bar">
<ul key="nav">
<li>
<Link className="link" to="/">
Home
</Link>
</li>
</ul>
</div>
Have encountered the same issue in my asp.net project, in the end i found the issue is with the target function not static, the issue fixed after I put the keyword static.
[WebMethod]
public static List<string> getRawData()
You can use this small library: https://github.com/ledfusion/php-rest-curl
Making a call is as simple as:
// GET
$result = RestCurl::get($URL, array('id' => 12345678));
// POST
$result = RestCurl::post($URL, array('name' => 'John'));
// PUT
$result = RestCurl::put($URL, array('$set' => array('lastName' => "Smith")));
// DELETE
$result = RestCurl::delete($URL);
And for the $result variable:
Hope it helps
As far as I know it is '
but it seems yours works as well
Uncaught TypeError: undefined is not a function example_app.js:7
This error message tells the whole story. On this line, you are trying to execute a function. However, whatever is being executed is not a function! Instead, it's undefined
.
So what's on example_app.js
line 7? Looks like this:
var tasks = new ExampleApp.Collections.Tasks(data.tasks);
There is only one function being run on that line. We found the problem! ExampleApp.Collections.Tasks
is undefined
.
So lets look at where that is declared:
var Tasks = Backbone.Collection.extend({
model: Task,
url: '/tasks'
});
If that's all the code for this collection, then the root cause is right here. You assign the constructor to global variable, called Tasks
. But you never add it to the ExampleApp.Collections
object, a place you later expect it to be.
Change that to this, and I bet you'd be good.
ExampleApp.Collections.Tasks = Backbone.Collection.extend({
model: Task,
url: '/tasks'
});
See how important the proper names and line numbers are in figuring this out? Never ever regard errors as binary (it works or it doesn't). Instead read the error, in most cases the error message itself gives you the critical clues you need to trace through to find the real issue.
In Javascript, when you execute a function, it's evaluated like:
expression.that('returns').aFunctionObject(); // js
execute -> expression.that('returns').aFunctionObject // what the JS engine does
That expression can be complex. So when you see undefined is not a function
it means that expression did not return a function object. So you have to figure out why what you are trying to execute isn't a function.
And in this case, it was because you didn't put something where you thought you did.
Try this
myApp.config(['$httpProvider', function($httpProvider) {
$httpProvider.defaults.useXDomain = true;
delete $httpProvider.defaults.headers.common['X-Requested-With'];
}
]);
Just setting useXDomain = true is not enough. AJAX request are also send with the X-Requested-With header, which indicate them as being AJAX. Removing the header is necessary, so the server is not rejecting the incoming request.
Lets say in scripts you want to run 2 commands with a single command:
"scripts":{
"start":"any command",
"singleCommandToRunTwoCommand":"some command here && npm start"
}
Now go to your terminal and run there npm run singleCommandToRunTwoCommand
.
I don't have enough rep here to add this as a comment, but IMO it's good style to write your headers with #include guards anyway, which as noted by Paranaix a few hours ago would prevent a multiple-definition error. Unless you're already using a separate CPP file, it's not necessary to use one just to initialize static non-integral members.
#ifndef FOO_H
#define FOO_H
#include "bar.h"
class foo
{
private:
static bar i;
};
bar foo::i = VALUE;
#endif
I see no need to use a separate CPP file for this. Sure, you can, but there's no technical reason why you should have to.
I have been searching for years for a solution and found this post on how to print a footer that works on multiple pages without overlapping page content.
My requirement was IE8, so far I have found that this does not work in Chrome. [update]As of 1 March 2018, it works in Chrome as well
This example uses tables and the tfoot element by setting the css style:
tfoot {display: table-footer-group;}
I'm a little late to the party, but I have a solution that might work for some who read this. It's in javascript instead of php, but there's a valid reason for it.
Full disclosure, I wrote this plugin...
Anyways.
The approach I've gone with is to allow a user to "Opt-In" to their profanity filtering. Basically profanity will be allowed by default, but if my users don't want to read it, they don't have to. This also helps with the "l33t sp3@k" issue.
The concept is a simple jquery plugin that gets injected by the server if the client's account is enabling profanity filtering. From there, it's just a couple simple lines that blot out the swears.
Here's the demo page
https://chaseflorell.github.io/jQuery.ProfanityFilter/demo/
<div id="foo">
ass will fail but password will not
</div>
<script>
// code:
$('#foo').profanityFilter({
customSwears: ['ass']
});
</script>
result
*** will fail but password will not
There are 3 steps that needs to be run.
It is very interesting that this simplest usage is not clearly described anywhere. And i believe there is a danger, you should pay attention to the verified_email
parameter coming in the response. Because if I am not wrong it may yield fake emails to register your application. (This is just my interpretation, has a fair chance that I may be wrong!)
I find facebook's OAuth mechanics much much clearly described.
Maybe you should look into using something simple like a truecrypt volume for source code storage as that seems to be a concern of yours. You can create an encrypted file on a usb key or just encrypt the whole volume (provided the code will fit) so you can simply take the key with you at the end of the day.
To compile, you could then use something like PyInstaller or py2exe in order to create a stand-alone executable. If you really wanted to go the extra mile, look into a packer or compression utility in order to add more obfuscation. If none of these are an option, you could at least compile the script into bytecode so it isn't immediately readable. Keep in mind that these methods will merely slow someone trying to debug or decompile your program.
One more dict style clean syntax:
df["new_column"] = df.apply(lambda x: x["A"] * x["B"], axis = 1)
or,
df["new_column"] = df["A"] * df["B"]
If youR data was in A1:C100
then:
Excel - all versions
=SUMPRODUCT(--(A1:A100="M"),--(C1:C100="Yes"))
Excel - 2007 onwards
=COUNTIFS(A1:A100,"M",C1:C100,"Yes")
In PHP5, you should use the Document Object Model class instead. Example:
$domDoc = new DOMDocument;
$rootElt = $domDoc->createElement('root');
$rootNode = $domDoc->appendChild($rootElt);
$subElt = $domDoc->createElement('foo');
$attr = $domDoc->createAttribute('ah');
$attrVal = $domDoc->createTextNode('OK');
$attr->appendChild($attrVal);
$subElt->appendChild($attr);
$subNode = $rootNode->appendChild($subElt);
$textNode = $domDoc->createTextNode('Wow, it works!');
$subNode->appendChild($textNode);
echo htmlentities($domDoc->saveXML());
change Settings Manager >> Preferred Applications >> Utilities
<script>
var today = new Date;
document.getElementById('date').innerHTML= today.toDateString();
</script>
To "call" means to make a reference in your code to a function that is written elsewhere. This function "call" can be made to the standard Python library (stuff that comes installed with Python), third-party libraries (stuff other people wrote that you want to use), or your own code (stuff you wrote). For example:
#!/usr/env python
import os
def foo():
return "hello world"
print os.getlogin()
print foo()
I created a function called "foo" and called it later on with that print statement. I imported the standard "os" Python library then I called the "getlogin" function within that library.
There are a number of dictionary files available online - if you're on linux, a lot of (all?) distros come with an /etc/dictionaries-common/words file, which you can easily parse (words = open('/etc/dictionaries-common/words').readlines()
, eg) for use.
When a clearfix is used in a parent container, it automatically wraps around all the child elements.
It is usually used after floating elements to clear the float layout.
When float layout is used, it will horizontally align the child elements. Clearfix clears this behaviour.
Example - Bootstrap Panels
In bootstrap, when the class panel is used, there are 3 child types: panel-header, panel-body, panel-footer. All of which have display:block layout but panel-body has a clearfix pre-applied. panel-body is a main container type whereas panel-header & panel-footer isn't intended to be a container, it is just intended to hold some basic text.
If floating elements are added, the parent container does not get wrapped around those elements because the height of floating elements is not inherited by the parent container.
So for panel-header & panel-footer, clearfix is needed to clear the float layout of elements: Clearfix class gives a visual appearance that the height of the parent container has been increased to accommodate all of its child elements.
<div class="container">
<div class="panel panel-default">
<div class="panel-footer">
<div class="col-xs-6">
<input type="button" class="btn btn-primary" value="Button1">
<input type="button" class="btn btn-primary" value="Button2">
<input type="button" class="btn btn-primary" value="Button3">
</div>
</div>
</div>
<div class="panel panel-default">
<div class="panel-footer">
<div class="col-xs-6">
<input type="button" class="btn btn-primary" value="Button1">
<input type="button" class="btn btn-primary" value="Button2">
<input type="button" class="btn btn-primary" value="Button3">
</div>
<div class="clearfix"/>
</div>
</div>
</div>
You can Simply use static variable to store the string of your edittext and then use that variable in the other class. Hope this will solve your problem
You can also specify the type of attachment you want in your e-mail, as an example I used pdf:
def send_email_pdf_figs(path_to_pdf, subject, message, destination, password_path=None):
## credits: http://linuxcursor.com/python-programming/06-how-to-send-pdf-ppt-attachment-with-html-body-in-python-script
from socket import gethostname
#import email
from email.mime.application import MIMEApplication
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
import smtplib
import json
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
with open(password_path) as f:
config = json.load(f)
server.login('[email protected]', config['password'])
# Craft message (obj)
msg = MIMEMultipart()
message = f'{message}\nSend from Hostname: {gethostname()}'
msg['Subject'] = subject
msg['From'] = '[email protected]'
msg['To'] = destination
# Insert the text to the msg going by e-mail
msg.attach(MIMEText(message, "plain"))
# Attach the pdf to the msg going by e-mail
with open(path_to_pdf, "rb") as f:
#attach = email.mime.application.MIMEApplication(f.read(),_subtype="pdf")
attach = MIMEApplication(f.read(),_subtype="pdf")
attach.add_header('Content-Disposition','attachment',filename=str(path_to_pdf))
msg.attach(attach)
# send msg
server.send_message(msg)
inspirations/credits to: http://linuxcursor.com/python-programming/06-how-to-send-pdf-ppt-attachment-with-html-body-in-python-script
You have three option here for removing duplicate item in your List:
Distinct(new DistinctItemComparer())
as @Christian Hayter mentioned.Use GroupBy
, but please note in GroupBy
you should Group by all of the columns because if you just group by Id
it doesn't remove duplicate items always. For example consider the following example:
List<Item> a = new List<Item>
{
new Item {Id = 1, Name = "Item1", Code = "IT00001", Price = 100},
new Item {Id = 2, Name = "Item2", Code = "IT00002", Price = 200},
new Item {Id = 3, Name = "Item3", Code = "IT00003", Price = 150},
new Item {Id = 1, Name = "Item1", Code = "IT00001", Price = 100},
new Item {Id = 3, Name = "Item3", Code = "IT00003", Price = 150},
new Item {Id = 3, Name = "Item3", Code = "IT00004", Price = 250}
};
var distinctItems = a.GroupBy(x => x.Id).Select(y => y.First());
The result for this grouping will be:
{Id = 1, Name = "Item1", Code = "IT00001", Price = 100}
{Id = 2, Name = "Item2", Code = "IT00002", Price = 200}
{Id = 3, Name = "Item3", Code = "IT00003", Price = 150}
Which is incorrect because it considers {Id = 3, Name = "Item3", Code = "IT00004", Price = 250}
as duplicate. So the correct query would be:
var distinctItems = a.GroupBy(c => new { c.Id , c.Name , c.Code , c.Price})
.Select(c => c.First()).ToList();
3.Override Equal
and GetHashCode
in item class:
public class Item
{
public int Id { get; set; }
public string Name { get; set; }
public string Code { get; set; }
public int Price { get; set; }
public override bool Equals(object obj)
{
if (!(obj is Item))
return false;
Item p = (Item)obj;
return (p.Id == Id && p.Name == Name && p.Code == Code && p.Price == Price);
}
public override int GetHashCode()
{
return String.Format("{0}|{1}|{2}|{3}", Id, Name, Code, Price).GetHashCode();
}
}
Then you can use it like this:
var distinctItems = a.Distinct();
//I think this way we could go :
int my_atoi(const char* snum)
{
int nInt(0);
int index(0);
while(snum[index])
{
if(!nInt)
nInt= ( (int) snum[index]) - 48;
else
{
nInt = (nInt *= 10) + ((int) snum[index] - 48);
}
index++;
}
return(nInt);
}
int main()
{
printf("Returned number is: %d\n", my_atoi("676987"));
return 0;
}
The most efficient, but not the C++ way would be:
FILE* f = fopen(filename, "r");
// Determine file size
fseek(f, 0, SEEK_END);
size_t size = ftell(f);
char* where = new char[size];
rewind(f);
fread(where, sizeof(char), size, f);
delete[] where;
#
EDIT - 2Just tested the std::filebuf
variant also. Looks like it can be called the best C++ approach, even though it's not quite a C++ approach, but more a wrapper. Anyway, here is the chunk of code that works almost as fast as plain C does.
std::ifstream file(filename, std::ios::binary);
std::streambuf* raw_buffer = file.rdbuf();
char* block = new char[size];
raw_buffer->sgetn(block, size);
delete[] block;
I've done a quick benchmark here and the results are following. Test was done on reading a 65536K binary file with appropriate (std::ios:binary
and rb
) modes.
[==========] Running 3 tests from 1 test case.
[----------] Global test environment set-up.
[----------] 4 tests from IO
[ RUN ] IO.C_Kotti
[ OK ] IO.C_Kotti (78 ms)
[ RUN ] IO.CPP_Nikko
[ OK ] IO.CPP_Nikko (106 ms)
[ RUN ] IO.CPP_Beckmann
[ OK ] IO.CPP_Beckmann (1891 ms)
[ RUN ] IO.CPP_Neil
[ OK ] IO.CPP_Neil (234 ms)
[----------] 4 tests from IO (2309 ms total)
[----------] Global test environment tear-down
[==========] 4 tests from 1 test case ran. (2309 ms total)
[ PASSED ] 4 tests.
Thanks for the tip Rodger.
For me it worked as below:
@echo off
start /d "" IEXPLORE.EXE www.google.com
start /d "" IEXPLORE.EXE www.yahoo.com
With the settings in Internet Explorer 8:
Use proper escaping: string.split("\\|")
Or, in Java 5+, use the helper Pattern.quote()
which has been created for exactly this purpose:
string.split(Pattern.quote("|"))
which works with arbitrary input strings. Very useful when you need to quote / escape user input.
You could to this trick: create a file 1 hour ago, and use the -newer file
argument.
(Or use touch -t
to create such a file).
zero and null are implicit pointers. If you arn't doing arithmetic, comparing, or printing '0' to screen there is no need to actually type it. Its implicit. As in implied. Typeof is also not required for the same reason. Watch.
if(obj) console.log("exists");
I didn't see request for a not or else there for it is not included as. As much as i love extra content which doesn't fit into the question. Lets keep it simple.
You can use something like this.
var {height, width} = Dimensions.get('window'); var textFontSize = width * 0.03;
inputText: {
color : TEXT_COLOR_PRIMARY,
width: '80%',
fontSize: textFontSize
}
Hope this helps without installing any third party libraries.
Using the BCNF definition
If and only if for every one of its dependencies X ? Y, at least one of the following conditions hold:
and the 3NF definition
If and only if, for each of its functional dependencies X ? A, at least one of the following conditions holds:
whereas
Where
That is, no partial subset (any non trivial subset except the full set) of a candidate key can be functionally dependent on anything other than a superkey.
A table/relation not in BCNF is subject to anomalies such as the update anomalies mentioned in the pizza example by another user. Unfortunately,
An example of the difference can currently be found at "3NF table not meeting BCNF (Boyce–Codd normal form)" on Wikipedia, where the following table meets 3NF but not BCNF because "Tennis Court" (a partial key/prime attribute) depends on "Rate Type" (a partial key/prime attribute that is not a superkey), which is a dependency we could determine by asking the clients of the database, the tennis club:
Today's Tennis Court Bookings (3NF, not BCNF)
Court Start Time End Time Rate Type
------- ---------- -------- ---------
1 09:30 10:30 SAVER
1 11:00 12:00 SAVER
1 14:00 15:30 STANDARD
2 10:00 11:30 PREMIUM-B
2 11:30 13:30 PREMIUM-B
2 15:00 16:30 PREMIUM-A
The table's superkeys are:
S1 = {Court, Start Time}
S2 = {Court, End Time}
S3 = {Rate Type, Start Time}
S4 = {Rate Type, End Time}
S5 = {Court, Start Time, End Time}
S6 = {Rate Type, Start Time, End Time}
S7 = {Court, Rate Type, Start Time}
S8 = {Court, Rate Type, End Time}
ST = {Court, Rate Type, Start Time, End Time}, the trivial superkey
The 3NF problem: The partial key/prime attribute "Court" is dependent on something other than a superkey. Instead, it is dependent on the partial key/prime attribute "Rate Type". This means that the user must manually change the rate type if we upgrade a court, or manually change the court if wanting to apply a rate change.
(In technical terms, we cannot guarantee that the "Rate Type" -> "Court" functional dependency will not be violated.)
The BCNF solution: If we want to place the above table in BCNF we can decompose the given relation/table into the following two relations/tables (assuming we know that the rate type is dependent on only the court and membership status, which we could discover by asking the clients of our database, the owners of the tennis club):
Rate Types (BCNF and the weaker 3NF, which is implied by BCNF)
Rate Type Court Member Flag
--------- ----- -----------
SAVER 1 Yes
STANDARD 1 No
PREMIUM-A 2 Yes
PREMIUM-B 2 No
Today's Tennis Court Bookings (BCNF and the weaker 3NF, which is implied by BCNF)
Member Flag Court Start Time End Time
----------- ----- ---------- --------
Yes 1 09:30 10:30
Yes 1 11:00 12:00
No 1 14:00 15:30
No 2 10:00 11:30
No 2 11:30 13:30
Yes 2 15:00 16:30
Problem Solved: Now if we upgrade the court we can guarantee the rate type will reflect this change, and we cannot charge the wrong price for a court.
(In technical terms, we can guarantee that the functional dependency "Rate Type" -> "Court" will not be violated.)
My comments on Renaud Bompuis's answer messed up.
Actually, you can use late binding, and the reference to the 11.0 object library is not required.
The following code will work without any references:
Dim f As Object
Set f = Application.FileDialog(3)
f.AllowMultiSelect = True
f.Show
MsgBox "file choosen = " & f.SelectedItems.Count
Note that the above works well in the runtime also.
Use clear: both;
I spent over a week trying to figure this out!
You can generate scripts to a file via SQL Server Management Studio, here are the steps:
When generating the scripts, there is an area that will allow you to script, constraints, keys, etc. From SQL Server 2008 R2 there is an Advanced Option under scripting:
>>> s1 ='arbit'
>>> s2 = 'hello world '.join( [s]*3 )
>>> print s2
arbit hello world arbit hello world arbit
private String message;
private ScreenManager s;
//Here is an example of code to add the keyListener() as suggested; modify
public void init(){
Window w = s.getFullScreenWindow();
w.addKeyListener(this);
public void keyPressed(KeyEvent e){
int keyCode = e.getKeyCode();
if(keyCode == KeyEvent.VK_F5)
message = "Pressed: " + KeyEvent.getKeyText(keyCode);
}
Before I get to my reiterated answer; I am confessing that the only answer I would accept here is this one by KM. above. I down voted the other answers because none of them actually answered the question asked or they were not adequate. PRINT output does indeed show up in the Message window, but that is not what was asked at all.
Why doesn't the PRINT statement output show during my Stored Procedure execution?
The short version of this answer is that you are sending your sproc's execution over to the SQL server and it isn't going to respond until it is finished with the whole transaction. Here is a better answer located at this external link.
Don't do these things
Alternative to PRINT inside of a Stored Procedure
Really this is kind of an icky work around in my opinion because the syntax is confusing in the context that it is being used in, but who knows maybe it will be updated in the future by Microsoft. I just don't like the idea of raising an error for the sole purpose of printing out debug info...
It seems like the only way around this issue is to use, as has been explained numerous times already RAISERROR WITH NOWAIT. I am providing an example and pointing out a small problem with this approach:
ALTER
--CREATE
PROCEDURE [dbo].[PrintVsRaiseErrorSprocExample]
AS
BEGIN
SET NOCOUNT ON;
-- This will print immediately
RAISERROR ('RE Start', 0, 1) WITH NOWAIT
SELECT 1;
-- Five second delay to simulate lengthy execution
WAITFOR DELAY '00:00:05'
-- This will print after the five second delay
RAISERROR ('RE End', 0, 1) WITH NOWAIT
SELECT 2;
END
GO
EXEC [dbo].[PrintVsRaiseErrorSprocExample]
Both SELECT statement results will only show after the execution is finished and the print statements will show in the order shown above.
Potential problem with this approach
Let's say you have both your PRINT statement and RAISERROR statement one after the other, then they both print. I'm sure this has something to do with buffering, but just be aware that this can happen.
ALTER
--CREATE
PROCEDURE [dbo].[PrintVsRaiseErrorSprocExample2]
AS
BEGIN
SET NOCOUNT ON;
-- Both the PRINT and RAISERROR statements will show
PRINT 'P Start';
RAISERROR ('RE Start', 0, 1) WITH NOWAIT
SELECT 1;
WAITFOR DELAY '00:00:05'
-- Both the PRINT and RAISERROR statements will show
PRINT 'P End'
RAISERROR ('RE End', 0, 1) WITH NOWAIT
SELECT 2;
END
GO
EXEC [dbo].[PrintVsRaiseErrorSprocExample2]
Therefore the work around here is, don't use both PRINT and RAISERROR, just choose one over the other. If you want your output to show during the execution of a sproc then use RAISERROR WITH NOWAIT.
You are correct in that glibc uses symbol versioning. If you are curious, the symbol versioning implementation introduced in glibc 2.1 is described here and is an extension of Sun's symbol versioning scheme described here.
One option is to statically link your binary. This is probably the easiest option.
You could also build your binary in a chroot build environment, or using a glibc-new => glibc-old cross-compiler.
According to the http://www.trevorpounds.com blog post Linking to Older Versioned Symbols (glibc), it is possible to to force any symbol to be linked against an older one so long as it is valid by using the same .symver
pseudo-op that is used for defining versioned symbols in the first place. The following example is excerpted from the blog post.
The following example makes use of glibc’s realpath, but makes sure it is linked against an older 2.2.5 version.
#include <limits.h>
#include <stdlib.h>
#include <stdio.h>
__asm__(".symver realpath,realpath@GLIBC_2.2.5");
int main()
{
const char* unresolved = "/lib64";
char resolved[PATH_MAX+1];
if(!realpath(unresolved, resolved))
{ return 1; }
printf("%s\n", resolved);
return 0;
}
Phoronix did some benchmarks about this, but it is about a snapshot version of Clang/LLVM from a few months back. The results being that things were more-or-less a push; neither GCC nor Clang is definitively better in all cases.
Since you'd use the latest Clang, it's maybe a little less relevant. Then again, GCC 4.6 is slated to have some major optimizations for Core 2 and i7, apparently.
I figure Clang's faster compilation speed will be nicer for original developers, and then when you push the code out into the world, Linux distro/BSD/etc. end-users will use GCC for the faster binaries.
Here is code for getting value return from Store procedure
Stored procedure:
alter proc [dbo].[UserlogincheckMVC]
@username nvarchar(max),
@password nvarchar(max)
as
begin
if exists(select Username from Adminlogin where Username =@username and Password=@password)
begin
return 1
end
else
begin
return 0
end
end
Code:
var parameters = new DynamicParameters();
string pass = EncrytDecry.Encrypt(objUL.Password);
conx.Open();
parameters.Add("@username", objUL.Username);
parameters.Add("@password", pass);
parameters.Add("@RESULT", dbType: DbType.Int32, direction: ParameterDirection.ReturnValue);
var RS = conx.Execute("UserlogincheckMVC", parameters, null, null, commandType: CommandType.StoredProcedure);
int result = parameters.Get<int>("@RESULT");
template<typename... Args>
std::string string_format(const char* fmt, Args... args)
{
size_t size = snprintf(nullptr, 0, fmt, args...);
std::string buf;
buf.reserve(size + 1);
buf.resize(size);
snprintf(&buf[0], size + 1, fmt, args...);
return buf;
}
Using C99 snprintf and C++11
This also one way of doing it and its works. Please check the following url https://jsfiddle.net/sarfarazk/ofgqm0sh/
<div class="container">
<div class="row">
<div class="col-md-6">
<div class="bg-success">Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
</div>
</div>
<div class="col-md-6">
<div class="bg-warning">Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium.
</div>
</div>
</div>
</div>
Another way of joining two lines without placing cursor to that line is:
:6,6s#\n##
Here 6 is the line number to which another line will be join. To display the line number, use :set nu
.
If we are on the cursor where the next line should be joined, then:
:s#\n##
In both cases we don't need g
like :s#\n##g
, because on one line only one \n
exist.
$('head').append('<meta http-equiv="X-UA-Compatible" content="IE=Edge" />');
or
var meta = document.createElement('meta');
meta.httpEquiv = "X-UA-Compatible";
meta.content = "IE=edge";
document.getElementsByTagName('head')[0].appendChild(meta);
Though I'm not certain it will have an affect as it will be generated after the page is loaded
If you want to add meta data tags for page description, use the SETTINGS of your DNN page to add Description and Keywords. Beyond that, the best way to go when modifying the HEAD is to dynamically inject your code into the HEAD via a third party module.
Found at http://www.dotnetnuke.com/Resources/Forums/forumid/7/threadid/298385/scope/posts.aspx
This may allow other meta tags, if you're lucky
Additional HEAD tags can be placed into Page Settings > Advanced Settings > Page Header Tags.
Found at http://www.dotnetnuke.com/Resources/Forums/forumid/-1/postid/223250/scope/posts.aspx
This is a simple way that works for me.
sudo ln -s /usr/bin/python3 /usr/bin/python
You could change /usr/bin/python3
for your path to python3 (or the version you want).
But keep in mind that update-alternatives
is probably the best choice.
ref: NSUserdefault objectTypes
Store
UserDefaults.standard.set(true, forKey: "Key") //Bool
UserDefaults.standard.set(1, forKey: "Key") //Integer
UserDefaults.standard.set("TEST", forKey: "Key") //setObject
Retrieve
UserDefaults.standard.bool(forKey: "Key")
UserDefaults.standard.integer(forKey: "Key")
UserDefaults.standard.string(forKey: "Key")
Remove
UserDefaults.standard.removeObject(forKey: "Key")
Remove all Keys
if let appDomain = Bundle.main.bundleIdentifier {
UserDefaults.standard.removePersistentDomain(forName: appDomain)
}
Store
NSUserDefaults.standardUserDefaults().setObject(newValue, forKey: "yourkey")
NSUserDefaults.standardUserDefaults().synchronize()
Retrieve
var returnValue: [NSString]? = NSUserDefaults.standardUserDefaults().objectForKey("yourkey") as? [NSString]
Remove
NSUserDefaults.standardUserDefaults().removeObjectForKey("yourkey")
registerDefaults: adds the registrationDictionary to the last item in every search list. This means that after NSUserDefaults has looked for a value in every other valid location, it will look in registered defaults, making them useful as a "fallback" value. Registered defaults are never stored between runs of an application, and are visible only to the application that registers them.
Default values from Defaults Configuration Files will automatically be registered.
for example detect the app from launch , create the struct for save launch
struct DetectLaunch {
static let keyforLaunch = "validateFirstlunch"
static var isFirst: Bool {
get {
return UserDefaults.standard.bool(forKey: keyforLaunch)
}
set {
UserDefaults.standard.set(newValue, forKey: keyforLaunch)
}
}
}
Register default values on app launch:
UserDefaults.standard.register(defaults: [
DetectLaunch.isFirst: true
])
remove the value on app termination:
func applicationWillTerminate(_ application: UIApplication) {
DetectLaunch.isFirst = false
}
and check the condition as
if DetectLaunch.isFirst {
// app launched from first
}
another one property suite name, mostly its used for App Groups concept, the example scenario I taken from here :
The use case is that I want to separate my UserDefaults (different business logic may require Userdefaults to be grouped separately) by an identifier just like Android's SharedPreferences. For example, when a user in my app clicks on logout button, I would want to clear his account related defaults but not location of the the device.
let user = UserDefaults(suiteName:"User")
use of userDefaults synchronize, the detail info has added in the duplicate answer.
Here are two scenarios where the keyup
event will not get fired:
Use the HTML5 input
event instead for a more robust solution:
<textarea maxlength='140'></textarea>
JavaScript (demo):
const textarea = document.querySelector("textarea");
textarea.addEventListener("input", event => {
const target = event.currentTarget;
const maxLength = target.getAttribute("maxlength");
const currentLength = target.value.length;
if (currentLength >= maxLength) {
return console.log("You have reached the maximum number of characters.");
}
console.log(`${maxLength - currentLength} chars left`);
});
And if you absolutely want to use jQuery:
$('textarea').on("input", function(){
var maxlength = $(this).attr("maxlength");
var currentLength = $(this).val().length;
if( currentLength >= maxlength ){
console.log("You have reached the maximum number of characters.");
}else{
console.log(maxlength - currentLength + " chars left");
}
});
Use
find \( -path "./tmp" -o -path "./scripts" \) -prune -o -name "*_peaks.bed" -print
or
find \( -path "./tmp" -o -path "./scripts" \) -prune -false -o -name "*_peaks.bed"
or
find \( -path "./tmp" -path "./scripts" \) ! -prune -o -name "*_peaks.bed"
The order is important. It evaluates from left to right. Always begin with the path exclusion.
Do not use -not
(or !
) to exclude whole directory. Use -prune
.
As explained in the manual:
-prune The primary shall always evaluate as true; it
shall cause find not to descend the current
pathname if it is a directory. If the -depth
primary is specified, the -prune primary shall
have no effect.
and in the GNU find manual:
-path pattern
[...]
To ignore a whole
directory tree, use -prune rather than checking
every file in the tree.
Indeed, if you use -not -path "./pathname"
,
find will evaluate the expression for each node under "./pathname"
.
find expressions are just condition evaluation.
\( \)
- groups operation (you can use -path "./tmp" -prune -o -path "./scripts" -prune -o
, but it is more verbose).-path "./script" -prune
- if -path
returns true and is a directory, return true for that directory and do not descend into it.-path "./script" ! -prune
- it evaluates as (-path "./script") AND (! -prune)
. It revert the "always true" of prune to always false. It avoids printing "./script"
as a match.-path "./script" -prune -false
- since -prune
always returns true, you can follow it with -false
to do the same than !
.-o
- OR operator. If no operator is specified between two expressions, it defaults to AND operator.Hence, \( -path "./tmp" -o -path "./scripts" \) -prune -o -name "*_peaks.bed" -print
is expanded to:
[ (-path "./tmp" OR -path "./script") AND -prune ] OR ( -name "*_peaks.bed" AND print )
The print is important here because without it is expanded to:
{ [ (-path "./tmp" OR -path "./script" ) AND -prune ] OR (-name "*_peaks.bed" ) } AND print
-print
is added by find - that is why most of the time, you do not need to add it in you expression. And since -prune
returns true, it will print "./script" and "./tmp".
It is not necessary in the others because we switched -prune
to always return false.
Hint: You can use find -D opt expr 2>&1 1>/dev/null
to see how it is optimized and expanded,
find -D search expr 2>&1 1>/dev/null
to see which path is checked.
Quickly ran a timeit to see, if there are any differences between the np.full
and np.ones
version.
Answer: No
import timeit
n_array, n_test = 1000, 10000
setup = f"import numpy as np; n = {n_array};"
print(f"np.ones: {timeit.timeit('np.ones((n, n), dtype=bool)', number=n_test, setup=setup)}s")
print(f"np.full: {timeit.timeit('np.full((n, n), True)', number=n_test, setup=setup)}s")
Result:
np.ones: 0.38416870904620737s
np.full: 0.38430388597771525s
IMPORTANT
Regarding the post about np.empty
(and I cannot comment, as my reputation is too low):
DON'T DO THAT. DON'T USE np.empty
to initialize an all-True
array
As the array is empty, the memory is not written and there is no guarantee, what your values will be, e.g.
>>> print(np.empty((4,4), dtype=bool))
[[ True True True True]
[ True True True True]
[ True True True True]
[ True True False False]]
Quoting directly from the help page for factor
:
To transform a factor f to its original numeric values, as.numeric(levels(f))[f]
is recommended and slightly more efficient than as.numeric(as.character(f))
.