Scribe.JS Lightweight Logger
I have looked through many loggers, and I wasn't able to find a lightweight solution - so I decided to make a simple solution that is posted on github.
- Saves the file which are organized by user, date, and level
- Gives you a pretty output (we all love that)
- Easy-to-use HTML interface
I hope this helps you out.
Online Demo
http://bluejamesbond.github.io/Scribe.js/
Secure Web Access to Logs

Prints Pretty Text to Console Too!
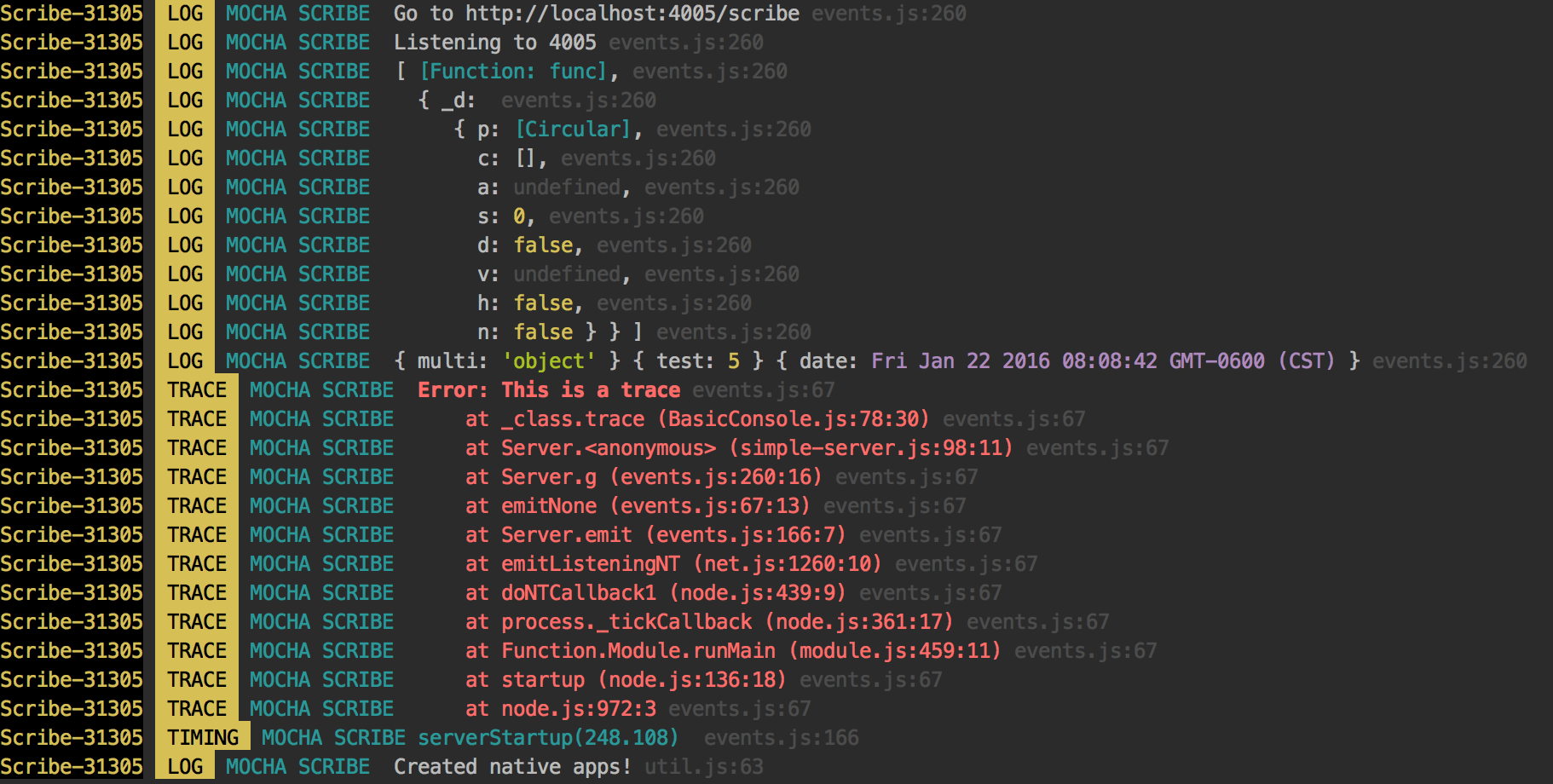
Web Access

Github
https://github.com/bluejamesbond/Scribe.js